no
/*
White space on empty fields
Start
*/
function clearWhiteSpaces() {
$("input").val(function (_, value) {
return $.trim(value);
});
}
/*
White space on empty fields
End
*/
/*
Utm parameters persistent on session
Start
*/
function findGetParameter(parameterName) {
var result = null,
tmp = [];
location.search
.substr(1)
.split("&")
.forEach(function (item) {
tmp = item.split("=");
if (tmp[0].toString().toLowerCase() === parameterName.toString().toLowerCase()) result = decodeURIComponent(tmp[1].toString().replace("%A0", ""));
});
return result;
}
function setutm() {
var r = findGetParameter("utm_campaign");
if (r) {
window.sessionStorage.setItem("utm_campaign", r);
}
r = findGetParameter("utm_content");
if (r) {
window.sessionStorage.setItem("utm_content", r);
}
r = findGetParameter("utm_medium");
if (r) {
window.sessionStorage.setItem("utm_medium", r);
}
r = findGetParameter("utm_source");
if (r) {
window.sessionStorage.setItem("utm_source", r);
}
r = findGetParameter("utm_term");
if (r) {
window.sessionStorage.setItem("utm_term", r);
}
}
function readutm() {
if ($("input[name='utm_campaign']").val() == "" && window.sessionStorage.getItem("utm_campaign")) {
$("input[name='utm_campaign']").val(window.sessionStorage.getItem("utm_campaign"));
}
if ($("input[name='utm_content']").val() == "" && window.sessionStorage.getItem("utm_content")) {
$("input[name='utm_content']").val(window.sessionStorage.getItem("utm_content"));
}
if ($("input[name='utm_medium']").val() == "" && window.sessionStorage.getItem("utm_medium")) {
$("input[name='utm_medium']").val(window.sessionStorage.getItem("utm_medium"));
}
if ($("input[name='utm_source']").val() == "" && window.sessionStorage.getItem("utm_source")) {
$("input[name='utm_source']").val(window.sessionStorage.getItem("utm_source"));
}
if ($("input[name='utm_term']").val() == "" && window.sessionStorage.getItem("utm_term")) {
$("input[name='utm_term']").val(window.sessionStorage.getItem("utm_term"));
}
}
function showFieldShared(currentField, col = false) {
var elem = $("[name=" + currentField + "]");
elem.parents(".form-element-layout").show();
if (col == "6") {
elem.closest(".grid-layout-col").addClass("col-md-6").removeClass("col-md-12");
}
if (col == "12") {
elem.closest(".grid-layout-col").addClass("col-md-12").removeClass("col-md-6");
}
}
function hideFieldShared(currentField) {
var elem = $("[name=" + currentField + "]");
elem.parents(".form-element-layout").hide();
}
if (typeof LeadRouting === "undefined") {
LeadRouting = "";
}
function checkTranslationThankYou() {
if (typeof translateLanguage != "undefined") {
translateLangThankYou = translateLanguage;
}
var tempLang = findGetParameter("tr_lang");
if (tempLang) {
translateLangThankYou = tempLang;
}
if (translateLangThankYou) {
$(".translate").each(function (i, element) {
element.innerHTML = translateThankYou(element.innerHTML.trim());
});
}
}
function translateThankYou(textToTranslate) {
var found = false;
if (typeof translateLangThankYou != "undefined" && typeof thankYouTranslations[textToTranslate] != "undefined" && typeof thankYouTranslations[textToTranslate][translateLangThankYou] != "undefined" && thankYouTranslations[textToTranslate][translateLangThankYou] != "") {
found = true;
textToTranslate = thankYouTranslations[textToTranslate][translateLangThankYou];
}
if (!found) {
translationsNotFoundThankYou.push(textToTranslate);
}
return textToTranslate;
}
var extraValidators = [];
function initializeExtraValidators() {
extraValidators = [];
if (typeof customExtraValidators == "undefined") {
customExtraValidators = [];
}
for (var validatorIndex = 0; validatorIndex < customExtraValidators.length; validatorIndex++) {
if (typeof customExtraValidators[validatorIndex] == "function") {
extraValidators.push(customExtraValidators[validatorIndex]);
customExtraValidators[validatorIndex](true);
}
}
if (typeof blackListValidatorEnabled != "undefined" && blackListValidatorEnabled == "true") {
extraValidators.push(blackListValidator);
blackListValidator(true);
}
if (typeof studentValidatorEnabled != "undefined" && studentValidatorEnabled == "true") {
extraValidators.push(studentValidator);
studentValidator(true);
}
if (extraValidators.length != 0) {
console.log("Extra validators", extraValidators);
$("form:not([name=translationPicklistForm])").submit(function (event) {
var extraValidatorsRejected = false;
for (var validatorIndex = 0; validatorIndex < extraValidators.length; validatorIndex++) {
if (!extraValidators[validatorIndex]()) {
console.log("Extra validator rejected " + extraValidators[validatorIndex].name);
extraValidatorsRejected = true;
}
else {
console.log("Extra validator passed " + extraValidators[validatorIndex].name);
}
}
if (extraValidatorsRejected) {
return false;
}
return true;
});
}
}
function studentValidator(init) {
if (typeof init != "undefined" && init) {
console.log("Extra validator student init");
if (typeof studentValidatorPopUpText !== "undefined") {
$("#studentPopUpValidatorText").text("");
if (typeof _translateRegistrationForm == "function") {
studentValidatorPopUpText = _translateRegistrationForm(studentValidatorPopUpText);
}
$("#studentPopUpValidatorText").append(studentValidatorPopUpText);
}
$(document).on("change", "[name='primaryRole'],[name='primaryRole1'],[name='primaryRole2']", function () {
studentValidator();
});
return;
}
if ($("[name='primaryRole']").val() == "Student" || $("[name='primaryRole1']").val() == "Student" || $("[name='primaryRole2']").val() == "Student") {
$("#popupStudentCommon").show();
$("#popupStudentCommonClose").click(function () {
$("#popupStudentCommon").hide();
$("[name='primaryRole'],[name='primaryRole1'],[name='primaryRole2']").val("");
});
return false;
}
return true;
}
if (typeof emailSubmitDisabled === "undefined") {
emailSubmitDisabled = false;
}
function blackListValidator(init) {
if (typeof init != "undefined" && init) {
console.log("Extra validator black list init");
$(document).on("change", "[name='emailAddress']", function () {
if (!emailSubmitDisabled) {
$("[type=Submit]").attr("disabled", false);
$("[type=Submit]").css("cursor", "pointer");
}
$(".loader").remove();
blackListValidator();
});
$(document).on("keyup", "[name='emailAddress']", function () {
if (!emailSubmitDisabled) {
$("[type=Submit]").attr("disabled", false);
$("[type=Submit]").css("cursor", "pointer");
}
$(".loader").remove();
blackListValidator();
});
$(document).on("keydown", "[name='emailAddress']", function () {
if (!emailSubmitDisabled) {
$("[type=Submit]").attr("disabled", false);
$("[type=Submit]").css("cursor", "pointer");
}
$(".loader").remove();
blackListValidator();
});
return;
}
$(".BlackListError").remove();
$("[name='emailAddress']").parent().removeClass("LV_invalid_field");
if (typeof emailBlacklist == "undefined") {
return true;
}
var exceptionMail = $("[name='emailAddress']").val();
var exceptionMailFound = emailBlacklistExceptions.find(function (i) {
return exceptionMail == i
});
if (exceptionMailFound) {
return true
}
splited = $("[name='emailAddress']").val().split("@");
if (splited.length > 1) {
splited[1] = splited[1].toLowerCase().trim();
var fullHost = splited[1];
host = fullHost.split(".")[0];
var lastPart = fullHost.split(".");
lastPart = lastPart[lastPart.length - 1];
const emailBlacklistfirstpart = [];
emailBlacklist.forEach(function (i) {
emailBlacklistfirstpart.push(i.split(".")[0]);
});
var exceptionFlag = true;
if (host == "mail") {
if (fullHost.includes(".ac.za") || ["us", "edu", "org", "mx", "ca", "sg"].includes(lastPart)) {
exceptionFlag = false;
}
}
if (exceptionFlag && emailBlacklistfirstpart.includes(host)) {
if (typeof emailBlacklistErrorMessage == "undefined") {
emailBlacklistErrorMessage = "Please provide your institution/business email address.";
}
temp = "<span style='display:block!important' class=\"BlackListError LV_validation_message LV_invalid\">" + emailBlacklistErrorMessage + "</span>";
$("[name='emailAddress']").parent().append(temp);
$("[name='emailAddress']").parent().addClass("LV_invalid_field");
return false;
}
}
return true;
}
/* Fix form height Start */
setInterval(function () {
if ($(document).width() >= 1200) {
$("#about").css("min-height", $("#download").height() - 140);
} else {
$("#about").css("min-height", "");
}
if (typeof fixInputHeights == "function") {
fixInputHeights();
}
}, 200);
/* Fix form height End */
var queryT = window.location.search.substring(1);
var varsT = queryT.split("&");
for (var i = 0; i < varsT.length; i++) {
var pair = varsT[i].split("=");
if (pair[0] == "debug") {
debug_mode = true;
}
if (pair[0] == "tr_lang") {
window.translateFormLanguage = pair[1];
}
if (pair[0] == "tr_lang_dates") {
window.datesLocale = pair[1];
}
}
if (typeof tr_lang !== "undefined" && tr_lang != "") {
window.translateFormLanguage = tr_lang;
}
if (typeof tr_lang_dates !== "undefined" && tr_lang_dates != "") {
window.datesLocale = tr_lang_dates;
}
// Initialization
setutm();
readutm();
clearWhiteSpaces();
showFieldShared("dietaryRequirements");
LeadRouting = LeadRouting.toLowerCase();
$('input[name="leadRouting"]').val(LeadRouting);
var emailBlacklist = [
"12yahoo.com",
"2005yahoo.com",
"44yahoo.com",
"6654.ki.com",
"96yahoo.com",
"975.hotmail.co.uk",
"adelphia.net",
"ameritech.net",
"aol.com",
"att.net",
"attglobal.net",
"ayahoo.com",
"bell.ca",
"bellsouth.com",
"bellsouth.net",
"bigpond.com",
"bigpond.net.au",
"blueyonder.co.uk",
"bt.com",
"btinternet.com",
"cable.comcast.com",
"carolina.rr.com",
"cfl.rr.com",
"charter.net",
"chotmail.com",
"comcast.net",
"cox.com",
"cox.net",
"csc.com",
"earthlink.net",
"email.com",
"excite.com",
"ft.hotmail.com",
"fuckhotmail.com",
"gmail.com",
"gmal.com",
"googlemail.com",
"hhotmail.com",
"home.com",
"hot.ee",
"hotmail.be",
"hotmail.bg",
"hotmail.ca",
"hotmail.ccom",
"hotmail.cim",
"hotmail.cmo",
"hotmail.co",
"hotmail.co.id",
"hotmail.co.il",
"hotmail.co.jp",
"hotmail.co.th",
"hotmail.co.uk",
"hotmail.co.za",
"hotmail.co0m",
"hotmail.coim",
"hotmail.cojm",
"hotmail.com",
"hotmail.com.ar",
"hotmail.com.au",
"hotmail.com.br",
"hotmail.com.mx",
"hotmail.com.my",
"hotmail.com.tr",
"hotmail.com.tw",
"hotmail.con",
"hotmail.conm",
"hotmail.cz",
"hotmail.de",
"hotmail.dk",
"hotmail.eg",
"hotmail.es",
"hotmail.ez",
"hotmail.fr",
"hotmail.gr",
"hotmail.hu",
"hotmail.it",
"hotmail.net",
"hotmail.nl",
"hotmail.om",
"hotmail.org",
"hotmail.ro",
"hotmail.rs",
"hotmail.ru",
"hotmail.se",
"hotmail.si",
"hotmail.uk",
"hotmaill.com",
"hotmailllllly.com",
"ieee.org",
"inbox.com",
"ix.netcom.com",
"jhotmail.com",
"juniper.net",
"juno.com",
"level3.com",
"live.ca",
"live.com",
"live.fr",
"lycos.com",
"mac.com",
"mail.com",
"mail.ru",
"mail.sprint.com",
"mail.yahoo.com",
"mailinator.com",
"mailyahoo.co.in",
"me.com",
"msn.com",
"nc.rr.com",
"ncmail.net",
"netscape.net",
"netzero.com",
"netzero.net",
"noemail.com",
"ntlworld.com",
"optonline.net",
"optusnet.com.au",
"pacbell.net",
"qwest.com",
"rediffmail.com",
"roadrunner.com",
"rocketmail.com",
"rogers.com",
"sbc.com",
"sbc.yahoo.com",
"sbcglobal.net",
"sbcyahoo.com",
"shaw.ca",
"sympatico.ca",
"tampabay.rr.com",
"telus.com",
"telus.net",
"tiscali.co.uk",
"twtelecom.com",
"tx.rr.com",
"tyahoo.com",
"verizon.com",
"verizon.net",
"verizonwireless.com",
"videotron.ca",
"webroot.com",
"wi.rr.com",
"worldnet.att.net",
"xtra.co.nz",
"yahoo.bom",
"yahoo.c",
"yahoo.ca",
"yahoo.ccom",
"yahoo.cim",
"yahoo.cm",
"yahoo.cn",
"yahoo.co",
"yahoo.co.id",
"yahoo.co.in",
"yahoo.co.jp",
"yahoo.co.kr",
"yahoo.co.nz",
"yahoo.co.th",
"yahoo.co.uk",
"yahoo.coin",
"yahoo.colin",
"yahoo.com",
"yahoo.com-",
"yahoo.com.ar",
"yahoo.com.au",
"yahoo.com.br",
"yahoo.com.cn",
"yahoo.com.hk",
"yahoo.com.mx",
"yahoo.com.my",
"yahoo.com.ph",
"yahoo.com.sg",
"yahoo.com.srg",
"yahoo.com.tr",
"yahoo.com.tw",
"yahoo.com.uk",
"yahoo.com.vn",
"yahoo.comm",
"yahoo.con",
"yahoo.coom",
"yahoo.cpm",
"yahoo.de",
"yahoo.dk",
"yahoo.es",
"yahoo.fr",
"yahoo.gr",
"yahoo.ie",
"yahoo.in",
"yahoo.it",
"yahoo.lt",
"yahoo.no",
"yahoo.ocm",
"yahoo.ocm.cn",
"yahoo.om",
"yahoo.pl",
"yahoo.se",
"yahooco.uk",
"yahool.co.th",
"yahool.com",
"yahoom.com",
"yahoomail.co.in",
"yahoomail.com",
"yahooo.com",
"ymail.com",
"aol.com",
"att.net",
"comcast.net",
"facebook.com",
"gmail.com",
"gmx.com",
"googlemail.com",
"google.com",
"hotmail.com",
"hotmail.co.uk",
"mac.com",
"me.com",
"mail.com",
"msn.com",
"live.com",
"sbcglobal.net",
"verizon.net",
"yahoo.com",
"yahoo.co.uk",
"email.com",
"games.com",
"gmx.net",
"hush.com",
"hushmail.com",
"icloud.com",
"inbox.com",
"lavabit.com",
"love.com",
"outlook.com",
"pobox.com",
"rocketmail.com",
"safe-mail.net",
"wow.com",
"ygm.com",
"ymail.com",
"zoho.com",
"fastmail.fm",
"yandex.com",
"bellsouth.net",
"charter.net",
"cox.net",
"earthlink.net",
"juno.com",
"btinternet.com",
"virginmedia.com",
"blueyonder.co.uk",
"freeserve.co.uk",
"live.co.uk",
"ntlworld.com",
"o2.co.uk",
"orange.net",
"sky.com",
"talktalk.co.uk",
"tiscali.co.uk",
"virgin.net",
"wanadoo.co.uk",
"bt.com",
"sina.com",
"qq.com",
"naver.com",
"hanmail.net",
"daum.net",
"nate.com",
"yahoo.co.jp",
"yahoo.co.kr",
"yahoo.co.id",
"yahoo.co.in",
"yahoo.com.sg",
"yahoo.com.ph",
"hotmail.fr",
"live.fr",
"laposte.net",
"yahoo.fr",
"wanadoo.fr",
"orange.fr",
"gmx.fr",
"sfr.fr",
"neuf.fr",
"free.fr",
"gmx.de",
"hotmail.de",
"live.de",
"online.de",
"t-online.de",
"web.de",
"yahoo.de",
"mail.ru",
"rambler.ru",
"yandex.ru",
"ya.ru",
"list.ru",
"hotmail.be",
"live.be",
"skynet.be",
"voo.be",
"tvcablenet.be",
"telenet.be",
"hotmail.com.ar",
"live.com.ar",
"yahoo.com.ar",
"fibertel.com.ar",
"speedy.com.ar",
"arnet.com.ar",
"yahoo.com.mx",
"live.com.mx",
"hotmail.es",
"hotmail.com.mx",
"prodigy.net.mx",
"29bg.freeserve.co.uk",
"abrownless.freeserve.co.uk",
"aipotu.freeserve.co.uk",
"appledown99.freeserve.co.uk",
"bauress.freeserve.co.uk",
"beth8082.freeserve.co.uk",
"cousins1.freeserve.co.uk",
"delucchi.freeseerve.co.uk",
"devlin-fitness.freeserve.co.uk",
"erldal97.freeserve.co.uk",
"free.fr",
"free.umobile.edu",
"free2Learn.org.uk",
"free411.com",
"free-fast-email.com",
"freelan.com.mx",
"freelance.com",
"freelance-idiomas.com.ar",
"freelancer.com",
"freemail.gr",
"freemail.hu",
"freemail.ru",
"freemymail.cz.cc",
"freenet.co.uk",
"freenet.de",
"freeolamail.com",
"free-q.com",
"free-qu.com",
"freesitemail.com",
"freeuk.com",
"freewave.com",
"msn.com",
"none.co",
"none.com",
"none.edu",
"noneofyourbusiness.com",
"nonsuchschool.org",
"onefreemail.co.cc",
"ostrycharz.free-online.co.uk",
"spamfree.thanks",
"stayfree.co.uk",
"textfree.us",
"theemailfree.com",
"yahoo.com",
"yars.free.net",
"ypd58.freeserve.co.uk",
"gmeal.com",
];
var emailBlacklistExceptions = [
"quintessa_hathaway@hotmail.com",
];
thankYouTranslations = {
Home: { nl: "Home", fr: "Accueil", sv: "Hem", es: "Inicio", pt: "Início", de: "Home", ar: "الرئيسية", tr: "Ana Sayfa" },
"About Blackboard": { nl: "Over Blackboard", fr: "A propos de Blackboard", sv: "Om Blackboard", es: "Acerca de Blackboard", pt: "Sobre o Blackboard", de: "Über Blackboard", ar: "حول بلاك بورد", tr: "Blackboard Hakkında" },
"About Anthology": { nl: "Over Anthology", fr: "A propos de Anthology", sv: "Om Anthology", es: "Acerca de Anthology", pt: "Sobre o Anthology", de: "Über Anthology", ar: "حول بلاك بورد", tr: "Anthology Hakkında" },
"Contact Us": { nl: "Contacteer ons", fr: "Nous contacter", sv: "Kontakta oss", es: "Contáctenos", pt: "Contato", de: "Kontakt", ar: "اتصل بنا", tr: "Bize Ulaşın" },
"Thank you for registering": { nl: "Uw registratie is bevestigd", fr: "Merci pour votre inscription", sv: "Tack för din anmälan", es: "Gracias por registrarse", pt: "Agradecemos a sua inscrição", de: "Vielen Dank für Ihre Registrierung", ar: "شكرًا لتسجيلك", tr: "Kayıt olduğunuz için teşekkürler" },
"Your registration for the webinar is confirmed.": { nl: "Uw deelname is bevestigd ", fr: "Votre inscription à notre webinaire est confirmée.", sv: "Din anmälan är bekräftad.", es: "A continuación encontrará los detalles de la sesión para los webinars.", pt: "Abaixo você encontrará os detalhes de cada sessão para os webinars.", de: "Wir bestätigen Ihre Registrierung für das Webinar.", ar: "تم تأكيد تسجيلك في الندوة عبر الويب.", tr: "Web seminerine kaydınız onaylandı." },
"Time &amp; Date:": { nl: "Tijd en Datum:", fr: "Heure et date:", sv: "Datum och tid:", es: "Fecha & hora:", pt: "Dia & hora :", de: "Datum ; Uhrzeit:", ar: "الوقت والتاريخ:", tr: "Zaman & Tarih:" },
"Time & Date:": { nl: "Tijd en Datum:", fr: "Heure et date:", sv: "Datum och tid:", es: "Fecha & hora:", pt: "Dia & hora :", de: "Datum ; Uhrzeit:", ar: "الوقت والتاريخ:", tr: "Zaman & Tarih:" },
"Your Time &amp; Date:": { nl: "Uw tijd en datum:", fr: "Heure et date locales:", sv: "Ditt datum och tid:", es: "Fecha & hora local:", pt: "Dia & hora local:", de: "Ortszeit:", ar: "التاريخ والوقت المحليين:", tr: "Senin zaman & Tarih:" },
"Your Time & Date:": { nl: "Uw tijd en datum:", fr: "Heure et date locales:", sv: "Ditt datum och tid:", es: "Fecha & hora local:", pt: "Dia & hora local:", de: "Ortszeit:", ar: "التاريخ والوقت المحليين:", tr: "Senin zaman & Tarih:" },
"Duration:": { nl: "Duurtijd:", fr: "Durée:", sv: "Längd:", es: "Duración:", pt: "Duração:", de: "Dauer:", ar: "المدة:", tr: "Süresi:" },
"minutes": { nl: "minuten", fr: "minutes", sv: "minuter", es: "minutos", pt: "minutos", de: "minuten", ar: "دقيقة", tr: "dakika" },
"View description »": { nl: "Bekijk beschrijving »", fr: "Voir Description »", sv: "Visa beskrivning »", es: "Ver descripción »", pt: "Ver Descrição »", de: "Beschreibung ansehen", ar: "عرض الوصف", tr: "Açıklamayı görüntüle" },
"Hide description »": { nl: "Verberg beschrijving »", fr: "Masquer description »", sv: "Dölj beskrivning »", es: "Ocultar descripción »", pt: "Ocultar descrição »", de: "Beschreibung verbergen", ar: "إخفاء الوصف", tr: "Açıklamayı gizle" },
"This webinar will take place using Blackboard Collaborate.": { nl: "Deze webinar wordt gegeven via Blackboard Collaborate.", fr: "Le webinaire se déroulera en salle de classe virtuelle Blackboard Collaborate.", sv: "Webinariet sker I webmötesverktyget Blackboard Collaborate.", es: "Se realizará el webinar a través de Blackboard Collaborate.", pt: "O webinar será realizado por meio do Blackboard Collaborate. A sala de Collaborate será aberta cerca de 10 minutos antes do horário de início.", de: "Das Webinar findet in einem virtuellen Konferenzraum von Blackboard Collaborate™ statt.", ar: "تعرض هذه الندوة عبر الويب باستخدام منصة Blackboard Collaborate.", tr: "Bu web semineri, Blackboard Collaborate kullanılarak gerçekleştirilecektir." },
"Learn how to get the best experience in using Blackboard Collaborate": { nl: "Ontdek hoe u de webinar het beste ervaart met Blackboard Collaborate", fr: "Apprenez comment bénéficier de la meilleure expérience Blackboard Collaborate", sv: "Bekanta dig med Blackboard Collaborate här", es: "Conozca como obtener una mejor experiencia usando Blackboard Collaborate", pt: "Use esse tempo para acessar e verificar se tudo funciona corretamente", de: "Besuchen Sie unsere Support-Seiten zur Nutzung von Blackboard Collaborate.", ar: "تعلّم كيف تستمتع بأفضل تجربة مع منصة Blackboard Collaborate.", tr: "Blackboard'u kullanırken en iyi deneyimi nasıl elde edeceğinizi öğrenin İşbirliği yap." },
"Add to Calendar": { nl: "Voeg toe aan de kalender", fr: "Ajouter au calendrier", sv: "Lägg till i kalendern", es: "GUARDAR EN CALENDARIO", pt: "Salvar no Calendário", de: "Ihrem Kalender hinzufügen", ar: "إضافة إلى التقويم", tr: "Takvime ekle" },
"Join the webinar": { nl: "Start de webinar", fr: "Rejoindre le webinaire", sv: "Gå med i webinaret", es: "Acceso al webinar", pt: "Acceso ao webinar", de: "Zum Webinar", ar: "انضم إلى الجلسة", tr: "Web seminerine katılın" },
"Sorry, we can't load your event details.": { nl: "Sorry, we kunnen uw evenement details niet laden.", fr: "Désolé, nous ne pouvons pas charger les détails de votre événement", sv: "Vi kan tyvärr inte ladda din evenemangsinformation.", es: "Lo sentimos, no podemos cargar los detalles de su evento.", pt: "Não foi possível enviar os detalhes do seu evento.", pt: "Lo sentimos, no podemos cargar los detalles de su evento.", de: "Sorry, wir können Ihre event-details nicht laden.", ar: "عذرًا، لا يمكننا تحميل تفاصيل حدثك.", tr: "Üzgünüz, etkinlik ayrıntılarınızı yükleyemiyoruz." },
"It seems you have an ad blocker in place or may have a browser which blocks cookies which prevents us from loading the event details.": { nl: "Het lijkt erop dat U een ad-blocker actief heeft of Uw webrowser blokkeert cookies waardoor wij de evenement details niet kunnen laden.", fr: "Il semble que vous ayez un bloqueur de publicité en place ou que vous ayez un navigateur qui bloque les cookies, ce qui nous empêche de charger les détails de l'événement.", sv: "Du verkar ha en annonsblockerare på plats eller en webbläsare som blockerar cookies som hindrar oss från att ladda händelsedetaljer.", es: "Lo sentimos, no podemos cargar los detalles de su evento. Parece que tenemos problemas para cargar los detalles de su evento. Inténtelo de nuevo. Si el problema persiste, contácte a hablecon@blackboard.com.", pt: "Parece que você tem um bloqueador de anúncios habilitado ou pode ter um navegador que bloqueia cookies, impedindo-nos de carregar os detalhes do evento.", de: "Leider können wir die Informationen zur Veranstaltung nicht laden. Dies kann passieren, wenn Ihre Verbindung langsam ist, wenn Ihr Browser keine Cookies akzeptiert oder wenn Sie ein Browser-Plugin zum Blockieren von Werbeanzeigen aktiviert haben.", ar: "يبدو أن لديك مانع إعلانات أو متصفح يحجب ملفات تعريف الارتباط مما يمنعنا من تحميل تفاصيل الحدث.", tr: "Görünüşe göre bir reklam engelleyiciniz var ya da olay ayrıntılarını yüklememizi engelleyen çerezleri engelleyen bir tarayıcınız olabilir." },
"Your registration has been received but please unblock this page and refresh so that we can show you the right links and details.": { nl: "We hebben uw registratie goed ontvangen, maar probeer deze pagina aan uw uitzonderingen toe te voegen van uw ad-blocker. Als U cookies geblokkeerd heeft in uw browser, voeg deze website dan toe aan uw uitzonderingen en laad de pagina opnieuw zodat wij de pagina correct kunnen weergeven.", fr: "Votre inscription a été reçue, mais veuillez débloquer cette page et actualiser afin que nous puissions vous partager les bons liens et détails.", sv: "Din registrering har mottagits. Vänligen avblockera den här sidan och uppdatera så att vi kan visa dig rätt länkar och detaljer.", es: "Se ha recibido su registro, sin embargo debe marcar esta página como desbloqueada y refrescar su navegador para que podamos mostrarle los enlaces y detalles correctos.", pt: "Seu registro foi recebido, no entanto, você deve marcar esta página como desbloqueada e atualizar seu navegador para que possamos mostrar os links e detalhes corretos.", de: "Bitte überprüfen Sie dies und versuchen Sie es erneut, indem Sie die Seite neu laden. Sollte das Problem bestehen bleiben, Setzen Sie sich bitte mit uns in Verbindung.", ar: "تم استلام تسجيلك ولكن يرجى فتح هذه الصفحة وتحديثها حتى نتمكن من عرض الروابط والتفاصيل الصحيحة.", tr: "Kaydınız alındı, ancak lütfen bu sayfanın engelini kaldırın ve size doğru bağlantıları ve ayrıntıları gösterebilmemiz için yenileyin." },
"That's embarrassing.": { nl: "Dit is vervelend.", fr: "C'est embarrassant.", sv: "Det här är lite pinsamt.", es: "¡Lo sentimos!", pt: "Sentimos muito!", de: "Das ist peinlich.", ar: "هذا محرج.", tr: "Bu utanç verici." },
"We seem to have trouble locating your event. Please try again.": { nl: "Het lijkt erop dat we problemen ondervinden om Uw evenement te vinden. Probeer het alstublieft opnieuw.", fr: "Nous semblons avoir du mal à localiser votre événement. Veuillez réessayer.", sv: "Vi har problem med att hitta ditt evenemang. Var god försök igen.", es: "Parece que tenemos problemas para cargar los detalles de su evento. Inténtelo de nuevo.", pt: "Parece que estamos tendo problemas para carregar os detalhes do seu evento. Tente de novo.", de: "Wir haben Probleme, Ihr webinar zu finden. Versuchs noch mal.", ar: "يبدو أن هناك مشكلة في تحديد موقع حدثك. يرجى المحاولة مرة أخرى.", tr: "Etkinliğinizi bulmakta sorun yaşıyoruz. Lütfen tekrar deneyin." },
"If the problem persists, please let us know at": { nl: " Als het probleem zich blijft voordoen, laat het ons weten via", fr: "Si le problème persiste, veuillez nous en informer en envoyant un email à", sv: " Om problemet kvarstår, vänligen meddela oss på", es: "Si el problema persiste, contácte a", pt: "Se o problema persistir, entre em contato", de: "Bitte überprüfen Sie den Link und versuchen Sie es erneut. Sollte das Problem bestehen bleiben, setzen Sie sich bitte mit uns in Verbindung", ar: "إذا استمرت المشكلة، يرجى التواصل معنا عبر", tr: "Sorun devam ederse, lütfen şu adresten bize bildirin" },
"One moment while we load the event details...": { nl: "Een ogenblik geduld alstublieft, we laden uw evenement…", fr: "Un instant pendant que nous chargeons les détails de l'événement…", sv: "Ett ögonblick medan vi laddar information om evenemanget…", es: "Un momento mientras encontramos tu registro…", pt: "Espere um momento enquanto carregamos os detalhes do seu treinamento…", pt: "Un momento mientras encontramos tu registro…", de: "Einen Moment, während wir die Veranstaltungsdetails laden…", ar: "لحظة من فضلك حتى نحمّل تفاصيل الحدث...", tr: "Etkinlik ayrıntılarını yüklerken bir dakika..." },
"At the time and date of the webinar, please use this link to join the session:": { nl: "Op de tijd en datum van de webinar, volg deze link om de sessie te starten:", fr: "À l'heure et à la date du webinaire, veuillez utiliser ce lien pour rejoindre la session:", sv: "Använd länken för att gå med i sessionen när webbinariet äger rum:", es: "En la fecha y hora del webinar, utilice este enlace para unirse a la sesión:", pt: "En la fecha y hora del webinar, utilice este enlace para unirse a la sesión:", de: "Zum Webinar:", ar: "في وقت وتاريخ الندوة عبر الويب، يرجى استخدام هذا الرابط للانضمام إلى الجلسة:", tr: "Webinarın yapılacağı saat ve tarihte, oturuma katılmak için lütfen şu bağlantıyı kullanın:" },
"We are looking forward to seeing you at our webinar!": { nl: "We kijken ernaaruit om U te ontmoeten tijdens onze webinar!", fr: "Nous avons hâte de vous voir lors de notre webinaire!", sv: "Vi ser fram emot att träffa dig på vårt webinar!", es: "¡Esperamos verlo en nuestro webinar!", pt: "¡Esperamos verlo en nuestro webinar!", de: "Wir freuen uns darauf, Sie in unserem Webinar begrüßen zu dürfen!", ar: "نحن نتطلع إلى رؤيتك في ندوة الويب الخاصة بنا!", tr: "Sizi web seminerimizde görmeyi dört gözle bekliyoruz!" },
"* Ensuring a good webinar experience *": { nl: "* Zo heeft u de beleefd U deze webinar het beste *", fr: "* Garantir une bonne experience de webinaire *", sv: "* För att allt ska flyta under webinariet *", es: "* Asegurando una buena experiencia de webinar *", pt: "* Garantindo uma boa experiência *", de: "* Gewährleistung einer guten Webinar-Erfahrung *", ar: "* ضمان تجربة جيدة للندوة عبر الويب *", tr: "* İyi bir web semineri deneyimi sağlamak *" },
"The webinar will take place in a Blackboard Collaborate&trade; virtual conference room. You will need to make sure you have a good internet connection and your computer speakers are turned on as audio will be via VOIP.": { nl: "The webinar vind plaats in een Blackboard Collaborate™ virtuele conferentie ruimte. Het is een vereiste dat U een goede en stabiele internetverbinding heeft en uw speakers van uw computer aan staan. De audio wordt gestreamd via VOIP.", fr: "Le webinaire aura lieu dans une salle de conférence virtuelle Blackboard Collaborate ™. Assurez-vous que vous disposez d'une bonne connexion Internet et que les haut-parleurs de votre ordinateur soient allumés car l'audio se fera via VOIP.", sv: "Webinariet sker I ett virtuellt konferensrum för Blackboard Collaborate ™. Säkerställ en bra internetanslutning och att datorhögtalarna är påslagna eftersom ljudet kommer via VOIP.", es: "El webinar tendrá lugar en una sala de conferencias virtual de Blackboard Collaborate&trade;. Asegúrese de tener una buena conexión a Internet y que los altavoces de su computadora estén encendidos ya que el audio se realizará a través de VOIP.", pt: "A sessão será realizada em uma sala de conferência virtual Blackboard Collaborate ™. Certifique-se de que tem uma boa ligação à Internet e de que os altifalantes do seu computador estão ligados, pois o áudio será via VOIP.", de: "Das Webinar findet in einem virtuellen Konferenzraum von Blackboard Collaborate™ statt. Stellen Sie sicher, dass Sie eine gute Internetverbindung haben und Ihre Computerlautsprecher eingeschaltet sind, da der Ton über VOIP übertragen wird. Für ein optimales Erlebnis empfehlen wir den Chrome Browser.", ar: "تعقد الندوة عبر الويب في غرفة اجتماعات افتراضية لـ Blackboard Collaborate. عليك التأكد من أن لديك اتصال إنترنت جيد وأن سماعات حاسوبك قيد التشغيل حيث سيكون الصوت من خلال وسيلة الصوت عبر بروتوكول الإنترنت VOIP.", tr: "Webinar, Blackboard Collaborate ™ sanal konferans odasında gerçekleştirilecektir. İyi bir internet bağlantınız olduğundan ve bilgisayar hoparlörlerinizin açık olduğundan emin olmanız gerekecek, çünkü ses VOIP aracılığıyla verilecektir." },
"The webinar will take place in a Blackboard Collaborate™ virtual conference room. You will need to make sure you have a good internet connection and your computer speakers are turned on as audio will be via VOIP.": { nl: "The webinar vind plaats in een Blackboard Collaborate™ virtuele conferentie ruimte. Het is een vereiste dat U een goede en stabiele internetverbinding heeft en uw speakers van uw computer aan staan. De audio wordt gestreamd via VOIP.", fr: "Le webinaire aura lieu dans une salle de conférence virtuelle Blackboard Collaborate ™. Assurez-vous que vous disposez d'une bonne connexion Internet et que les haut-parleurs de votre ordinateur soient allumés car l'audio se fera via VOIP.", sv: "Webinariet sker I ett virtuellt konferensrum för Blackboard Collaborate ™. Säkerställ en bra internetanslutning och att datorhögtalarna är påslagna eftersom ljudet kommer via VOIP.", es: "El webinar tendrá lugar en una sala de conferencias virtual de Blackboard Collaborate&trade;. Asegúrese de tener una buena conexión a Internet y que los altavoces de su computadora estén encendidos ya que el audio se realizará a través de VOIP.", pt: "A sessão será realizada em uma sala de conferência virtual Blackboard Collaborate ™. Certifique-se de que tem uma boa ligação à Internet e de que os altifalantes do seu computador estão ligados, pois o áudio será via VOIP.", de: "Das Webinar findet in einem virtuellen Konferenzraum von Blackboard Collaborate™ statt. Stellen Sie sicher, dass Sie eine gute Internetverbindung haben und Ihre Computerlautsprecher eingeschaltet sind, da der Ton über VOIP übertragen wird. Für ein optimales Erlebnis empfehlen wir den Chrome Browser.", ar: "تعقد الندوة عبر الويب في غرفة اجتماعات افتراضية لـ Blackboard Collaborate. عليك التأكد من أن لديك اتصال إنترنت جيد وأن سماعات حاسوبك قيد التشغيل حيث سيكون الصوت من خلال وسيلة الصوت عبر بروتوكول الإنترنت VOIP.", tr: "Webinar, Blackboard Collaborate ™ sanal konferans odasında gerçekleştirilecektir. İyi bir internet bağlantınız olduğundan ve bilgisayar hoparlörlerinizin açık olduğundan emin olmanız gerekecek, çünkü ses VOIP aracılığıyla verilecektir." },
"* Browser based *": { nl: "* Browser based *", fr: "* Navigateur *", sv: "* Webbläsarbaserat *", es: "* Baseado no navegador *", pt: "", de: "* Fenêtre de navigateur *", ar: "* تستند إلى المتصفح *", tr: "* Tarayıcı tabanlı *" },
"The webinar will be hosted in a browser window. We recommend using Chrome&trade; for the best experience.": { nl: "De webinar wordt gehost in een webbrowser sherm. Voor de beste ervaring adviseren wij U Chrome™ te gebruiken.", fr: "Le webinaire sera hébergé dans une fenêtre de navigateur. Nous vous recommandons d'utiliser Chrome ™ pour une expérience optimale.", sv: "Webinariet sker via webläsare. Vi rekommenderar Chrome ™ för den bästa upplevelsen.", es: "El webinar se ejecutará en una ventana de navegador. Recomendamos usar Chrome&trade; para una mejor experiencia.", pt: "A sessão será executada em uma janela do navegador. Recomendamos o uso do Chrome™ para uma melhor experiência.", de: "Für ein optimales Erlebnis empfehlen wir den Chrome Browser.", ar: "يتم استضافة الندوة عبر الويب في نافذة متصفح. نوصي باستخدام جوجل كروم للحصول على أفضل تجربة.", tr: "Webebinar bir tarayıcı penceresinde düzenlenecektir. En iyi deneyim için Chrome ™ kullanmanızı öneririz." },
"The webinar will be hosted in a browser window. We recommend using Chrome™ for the best experience.": { nl: "De webinar wordt gehost in een webbrowser sherm. Voor de beste ervaring adviseren wij U Chrome™ te gebruiken.", fr: "Le webinaire sera hébergé dans une fenêtre de navigateur. Nous vous recommandons d'utiliser Chrome ™ pour une expérience optimale.", sv: "Webinariet sker via webläsare. Vi rekommenderar Chrome ™ för den bästa upplevelsen.", es: "El webinar se ejecutará en una ventana de navegador. Recomendamos usar Chrome&trade; para una mejor experiencia.", pt: "A sessão será executada em uma janela do navegador. Recomendamos o uso do Chrome™ para uma melhor experiência.", de: "Für ein optimales Erlebnis empfehlen wir den Chrome Browser.", ar: "يتم استضافة الندوة عبر الويب في نافذة متصفح. نوصي باستخدام جوجل كروم للحصول على أفضل تجربة.", tr: "Webebinar bir tarayıcı penceresinde düzenlenecektir. En iyi deneyim için Chrome ™ kullanmanızı öneririz." },
"* Join Early *": { nl: "* Log in een paar minuten voor de webinar begint *", fr: "* Rejoindre la session en avance *", sv: "* Gå med tidigt *", es: "* Únase con tiempo *", pt: "* Junte-se cedo *", de: "* Details zur Teilnahme *", ar: "* انضم مبكرًا *", tr: "* Erken Katılın *" },
"If you can, join a session early and get to know your way around. You can then also set up your audio and video.": { nl: "", fr: "Si vous le pouvez, rejoignez une session 5-10 minutes avant l'heure prévue et familiarisez vous avec l'environement. Vous pouvez également configurer votre audio et vidéo.", sv: "Om du kan, gå med i sessionen lite tidigare för att bekanta dig. Också bra för att hinna ställa in ljud.", es: "De ser posible, únase a una sesión con tiempo. Posteriormente, también podrá configurar su audio y video", pt: "Se possível, junte-se a uma sessão mais cedo. Posteriormente, você também pode configurar seu áudio e vídeo", de: "Stellen Sie sicher, dass Sie den virtuellen Lernraum mindestens 5-10 Minuten vor Beginn Ihres Webinars betreten. Für ein perfektes Webinar-Erlebnis beachten Sie bitte unbedingt die folgenden Tipps.", ar: "إذا استطعت، انضم إلى الجلسة مبكرًا وتعرف على ما تريد. يمكنك بعد ذلك أيضًا إعداد الصوت والفيديو.", tr: "Mümkünse, erken bir oturuma katılın ve yolunuzu tanıyın. Daha sonra ses ve videonuzu da ayarlayabilirsiniz." },
"* Learn More *": { nl: "* Hulp nodig? *", fr: "* En savoir plus *", sv: "* Läs mer *", es: "* Conozca más *", pt: "* Saiba mais *", de: "* Mehr erfahren *", ar: "*تعلّم المزيد*", tr: "* Daha fazla bilgi edin *" },
"Follow this link to learn more:": { nl: "Klik op deze link om de help pagina op te reopen:", fr: "Suivez ce lien pour en savoir plus:", sv: "Webinariet sker I webmötesverktyget Blackboard Collaborate:", es: "Siga este enlace para obtener más información:", pt: "Siga este link para mais informações:", de: "Folgen Sie diesem Link, um mehr erfahren:", ar: "اتبع هذا الرابط لتعلّم المزيد:", tr: "Daha fazla bilgi edinmek için bu bağlantıyı izleyin" },
"Follow this link to learn more: ": { nl: "Klik op deze link om de help pagina op te reopen: ", fr: "Suivez ce lien pour en savoir plus: ", sv: "Webinariet sker I webmötesverktyget Blackboard Collaborate: ", es: "Siga este enlace para obtener más información: ", pt: "Siga este link para mais informações: ", de: "Folgen Sie diesem Link, um mehr erfahren: ", ar: "اتبع هذا الرابط لتعلّم المزيد: ", tr: "Daha fazla bilgi edinmek için bu bağlantıyı izleyin " },
"If you have any further questions about this webinar, the webinar series, or Blackboard in general, please send us an email at": { nl: "Mocht U meer vragen hebben over deze webinar, of onze andere webinar serie of algemene vragen over", fr: "Pour toute question concernant ce webinair, la série de webinaires ou Blackboard en général, n'hésitez pas à nous écrire à", sv: "Om du har fler frågor om webbinariet, webinarserien eller Blackboard i allmänhet, skicka ett e-postmeddelande till oss på", es: "Si tiene preguntas sobre este webinar, la serie de webinars o sobre Blackboard en general, por favor envíenos un email a", pt: "Si tiene preguntas sobre este webinar, la serie de webinars o sobre Blackboard en general, por favor envíenos un email a", de: "Wenn Sie weitere Fragen haben, senden Sie uns bitte eine E-Mail an", ar: "إذا كانت لديك أسئلة أخرى حول هذه الندوة عبر الويب أو سلسلة الندوة أو بلاك بورد بشكل عام، فيرجى إرسال بريد إلكتروني إلى", tr: "Bu webinarı, webinar serisi veya genel olarak Blackboard hakkında başka sorularınız varsa, lütfen şu adresten bize bir e-posta gönderin:" },
"AskUs@blackboard.com": { nl: "AskEUROPE@blackboard.com", fr: "AskEUROPE@blackboard.com", sv: "AskEUROPE@blackboard.com", es: "hablecon@blackboard.com", pt: "hablecon@blackboard.com", de: "AskEUROPE@blackboard.com", ar: "AskMea@blackboard.com", tr: "AskMea@blackboard.com" },
"If you have any further questions about this webinar, the webinar series, or Blackboard in general, please send us an email at ": { nl: "Mocht U meer vragen hebben over deze webinar, of onze andere webinar serie of algemene vragen over ", fr: "Pour toute question concernant ce webinair, la série de webinaires ou Blackboard en général, n'hésitez pas à nous écrire à ", sv: "Om du har fler frågor om webbinariet, webinarserien eller Blackboard i allmänhet, skicka ett e-postmeddelande till oss på ", es: "Si tiene preguntas sobre este webinar, la serie de webinars o sobre Blackboard en general, por favor envíenos un email a ", pt: "Si tiene preguntas sobre este webinar, la serie de webinars o sobre Blackboard en general, por favor envíenos un email a ", de: "Wenn Sie weitere Fragen haben, senden Sie uns bitte eine E-Mail an ", ar: "إذا كانت لديك أسئلة أخرى حول هذه الندوة عبر الويب أو سلسلة الندوة أو بلاك بورد بشكل عام، فيرجى إرسال بريد إلكتروني إلى ", tr: "Bu webinarı, webinar serisi veya genel olarak Blackboard hakkında başka sorularınız varsa, lütfen şu adresten bize bir e-posta gönderin " },
"Online using Blackboard Collaborate": { nl: "Deze webinar wordt gegeven via Blackboard Collaborate", fr: "En ligne via Blackboard Collaborate", sv: "Webinariet sker I webmötesverktyget Blackboard Collaborate", es: "En línea con Blackboard Collaborate", pt: "Online com o Blackboard Collaborate", de: "Online mit Blackboard Collaborate", ar: "باستخدام منصة Blackboard Collaborate", tr: "Blackboard Collaborate aracılığıyla çevrimiçi" },
"https://help.blackboard.com/Collaborate/Ultra/Participant/Get_Started": { nl: "https://help.blackboard.com/nl-nl/Collaborate/Ultra/Participant/Get_Started", fr: "https://help.blackboard.com/fr-fr/Collaborate/Ultra/Participant/Get_Started", sv: "https://help.blackboard.com/sv-se/Collaborate/Ultra/Participant/Get_Started", es: "https://help.blackboard.com/es-es/Collaborate/Ultra/Participant/Get_Started", pt: "https://help.blackboard.com/pt-br/Collaborate/Ultra/Participant/Get_Started", de: "https://help.blackboard.com/de-de/Collaborate/Ultra/Participant/Get_Started", ar: "https://help.blackboard.com/ar-sa/Collaborate/Ultra/Participant/Get_Started", tr: "https://help.blackboard.com/tr-tr/Collaborate/Ultra/Participant/Get_Started" },
"https://help.blackboard.com/Collaborate/Ultra/Participant": { nl: "https://help.blackboard.com/nl-nl/Collaborate/Ultra/Participant/", fr: "https://help.blackboard.com/fr-fr/Collaborate/Ultra/Participant/", sv: "https://help.blackboard.com/sv-se/Collaborate/Ultra/Participant/", es: "https://help.blackboard.com/es-es/Collaborate/Ultra/Participant/", pt: "https://help.blackboard.com/pt-br/Collaborate/Ultra/Participant/", de: "https://help.blackboard.com/de-de/Collaborate/Ultra/Participant/", ar: "https://help.blackboard.com/ar-sa/Collaborate/Ultra/Participant/", tr: "https://help.blackboard.com/tr-tr/Collaborate/Ultra/Participant/" },
};
thankYouTranslations["Thank You For Registering"] = thankYouTranslations["Thank you for registering"];
translationsNotFoundThankYou = [];
translateLangThankYou = "en";
initializeExtraValidators();
if (typeof translateRegistrationForm == "function") {
translateRegistrationForm();
}
function checkFurtherEducationBasedOnCountry() {
var country = $("select[name='country']").val();
country = (country + "").toString().toLowerCase();
if (["usa", "united states", "canada", "us", "ca"].includes(country)) {
$("[value='Further Education']").hide();
} else {
$("[value='Further Education']").show();
}
}
function checkOptInBasedOnCountry() {
var country = $("select[name='country']").val();
country = (country + "").toString().toLowerCase();
var lacCountries = ["Anguilla", "Antigua and Barbuda", "Argentina", "Aruba", "Barbados", "Belize", "Bermuda", "Bolivia", "Bonaire", "Cayman Islands", "Chile", "Colombia", "Costa Rica", "Jamaica", "Cuba", "Curacao", "Dominica", "Dominican Republic", "Ecuador", "El Salvador", "Falkland Islands (malvinas)", "French Guiana", "Grenada", "Guadeloupe", "Guatemala", "Guyana", "Haiti", "Honduras", "Martinique", "Mexico", "Montserrat", "Nicaragua", "Panama", "Paraguay", "Peru", "Saint Kitts and Nevis", "Saint Lucia", "Saint Martin", "St Vincent and the Grenadines", "Sth Georgia & Sth Sandwich Is", "Sth Georgia & Sth Sandwich Is", "Suriname", "The Bahamas", "Trinidad and Tobago", "Turks and Caicos Islands", "Uruguay", "Venezuela", "Virgin Islands (British)"];
for (var i = 0; i < lacCountries.length; i++) {
lacCountries[i] = (lacCountries[i] + "").toString().toLowerCase();
}
if (["usa", "united states", "us"].includes(country) || lacCountries.includes(country)) {
$("[name=OptIn]").prop("checked", true);
} else {
$("[name=OptIn]").prop("checked", false);
$("[name=OptIn]").closest(".layout-col.d-none").removeClass("d-none");
}
$("[name=OptIn]").trigger("change");
}
function initAreaOfInterestAutomation(region) {
var demo = typeof areaOfInterestAutomationDemo !== "undefined" && areaOfInterestAutomationDemo === "true";
if (region == "global") {
if (typeof document.getElementsByName("hiddenAreaOfInterestAutomation")[0] != "undefined") {
document.getElementsByName("hiddenAreaOfInterestAutomation")[0].value = "global";
}
var listBB = ["Blackboard", "Anthology Ally", "Online Program Experience (OPX)", "Research & Strategy", "Performance Marketing", "Enrollment Management", "One Stop", "Help Desk", "Retention Coaching", "Chatbot", "Consulting Services", "Managed Services", "Training"];
var listAnthology = ["Anthology Reach", "Anthology Digital Assistant", "Anthology Engage", "Anthology Milestone", "Anthology Occupation Insight",
"Anthology Encompass", "Anthology Raise", "Anthology Student", "Anthology Finance & HCM Management", /*"Anthology Human Capital Management",*/ "Anthology Payroll", "Anthology Student Verification",
"Anthology Insight", "Anthology Collective Review", "Anthology Evaluate", "Anthology Outcomes", "Anthology Planning", "Anthology Portfolio", "Anthology Accreditation",
"Anthology Program Review"];
var bbSelector = ["Accessibility & Inclusive Access", /*"Learning Effectiveness",*/ /*"Online Program Management",*/ /*"Recruitment",*/ "Strategic Marketing & Recruiting Services", "Support & Retention Services", "Teaching & Learning", "Consulting Services"];
var anthologySelector = ["Accreditation & Self-Study", "Alumni & Advancement", "Assessment Management", "Career Development", "Data & Analytics", "Enrollment & Retention", "Enterprise Performance", "Student Information & Enterprise Resources"];
var opValueMapping = {
"Accessibility & Inclusive Access": "Accessibility",
/*"Learning Effectiveness": "Learning Solutions",*/
/*Recruitment: "Strategic Marketing & Recruiting Services",*/
"Strategic Marketing & Recruiting Services": "Strategic Marketing & Recruiting Services",
"Support & Retention Services": "IT Help Desk & One Stop Services",
"Teaching & Learning": "Learning Solutions",
"Consulting Services": "Enterprise Consulting",
"Anthology Ally": "Blackboard Ally",
"Online Program Experience (OPX)": "Marketing & Enrollment Services",
"Research & Strategy": "Marketing & Enrollment Services",
"Performance Marketing": "Marketing & Enrollment Services",
"Enrollment Management": "Marketing & Enrollment Services",
"One Stop": "One Stop Services",
"Help Desk": "IT Help Desk Services",
"Retention Coaching": "Marketing & Enrollment Services",
"Chatbot": "IT Help Desk Services",
"Managed Services": "Consulting Services",
"Training": "Blackboard Academy",
};
var customSelector = {
"Accreditation & Self-Study": ["Anthology Accreditation", "Anthology Program Review"],
"Alumni & Advancement": ["Anthology Encompass", "Anthology Raise"],
"Assessment Management": ["Anthology Collective Review", "Anthology Evaluate", "Anthology Outcomes", "Anthology Planning", "Anthology Portfolio"],
"Career Development": ["Anthology Milestone", "Anthology Occupation Insight"],
"Enrollment & Retention": ["Anthology Reach", "Anthology Digital Assistant", "Anthology Engage", "Anthology Succeed", "Anthology Apply"],
"Enterprise Performance": ["Anthology Insight"],
"Student Information & Enterprise Resources": ["Anthology Student", "Anthology Finance & HCM", "Anthology Payroll", "Anthology Student Verification"],
"Accessibility & Inclusive Access": ["Anthology Ally"],
"Strategic Marketing & Recruiting Services": ["Online Program Experience (OPX)", "Research & Strategy", "Performance Marketing", "Enrollment Management", "Retention Coaching"],
"Support & Retention Services": ["One Stop", "Help Desk", "Chatbot"],
"Teaching & Learning": ["Blackboard", "Anthology Ally"],
"Consulting Services": ["Consulting Services", "Managed Services", "Training"],
"Data & Analytics": ["Anthology Illuminate"]
};
}
else if (region == "international") {
if (typeof document.getElementsByName("hiddenAreaOfInterestAutomation")[0] != "undefined") {
document.getElementsByName("hiddenAreaOfInterestAutomation")[0].value = "international";
}
var listBB = ["Anthology Ally", "Blackboard"];
var listAnthology = ["Anthology Evaluate", "Anthology Engage", "Anthology Milestone", "Anthology Portfolio", "Anthology Reach", "Anthology Student"];
var bbSelector = ["Accessibility & Inclusive Access", "Teaching & Learning", "Consulting Services"];
var anthologySelector = ["Assessment Management", "Career Development", "Data & Analytics", "Enrollment & Retention", /*"Learning Effectiveness",*/ "Student Information & Enterprise Resources"];
var opValueMapping = {
"Accessibility & Inclusive Access": "Accessibility",
/*"Learning Effectiveness" : "Learning Solutions",*/
"Recruitment": "Strategic Marketing & Recruiting Services",
"Support & Retention": "IT Help Desk & One Stop Services",
"Teaching & Learning": "Learning Solutions",
"Consulting Services": "Enterprise Consulting",
"Anthology Ally": "Blackboard Ally",
"Online Program Experience (OPX)": "Marketing & Enrollment Services",
"Research & Strategy": "Marketing & Enrollment Services",
"Performance Marketing": "Marketing & Enrollment Services",
"Enrollment Management": "Marketing & Enrollment Services",
"One Stop": "One Stop Services",
"Help Desk": "IT Help Desk Services",
"Retention Coaching": "Marketing & Enrollment Services",
"Chatbot": "IT Help Desk Services",
"Managed Services": "Consulting Services",
"Training": "Blackboard Academy",
};
var customSelector = {
"Assessment Management": ["Anthology Evaluate", "Anthology Portfolio"],
"Career Development": ["Anthology Milestone"],
"Enrollment & Retention": ["Anthology Engage", "Anthology Reach"],
"Student Information": ["Anthology Student"],
"Student Information & Enterprise Resources": ["Anthology Student"],
"Teaching & Learning": ["Blackboard", "Anthology Ally"],
"Accessibility & Inclusive Access": ["Anthology Ally"],
"Consulting Services": ["Consulting Services", "Managed Services", "Training"],
"Data & Analytics": ["Anthology Illuminate"]
};
}
else {
return;
}
var areaOfInterestJoined = [];
bbSelector.map(function (item) {
areaOfInterestJoined.push(item);
});
anthologySelector.map(function (item) {
areaOfInterestJoined.push(item);
});
areaOfInterestJoined.sort();
listBB.sort();
listAnthology.sort();
if ($("[name=areaofInterest1]").length > 0) {
var $areaofInterest = $("[name=areaofInterest1]");
}
else if ($("[name=areaofInterest]").length > 0) {
var $areaofInterest = $("[name=areaofInterest]");
}
else if ($("[name=areaOfInterest]").length > 0) {
var $areaofInterest = $("[name=areaOfInterest]");
}
else {
console.error("No area of interest field found");
return;
}
if ($("[name=productServiceInterest1]").length > 0) {
var $productServiceInterest = $("[name=productServiceInterest1]");
}
else if ($("[name=productServiceInterest]").length > 0) {
var $productServiceInterest = $("[name=productServiceInterest]");
} else {
console.error("No product service interest field found");
return;
}
if ($("[name=hiddenAreaOfInterest]").length > 0) {
var $hiddenAreaOfInterest = $("[name=hiddenAreaOfInterest]");
}
else {
console.error("No hidden area of interest field found");
return;
}
if ($("[name=hiddenProductServiceInterest1]").length > 0) {
var $hiddenProductServiceInterest = $("[name=hiddenProductServiceInterest1]");
}
else if ($("[name=hiddenProductServiceInterest]").length > 0) {
var $hiddenProductServiceInterest = $("[name=hiddenProductServiceInterest]");
}
else {
console.error("No hidden product service field found");
return;
}
var areaofInterestFieldValidation = new LiveValidation($areaofInterest[0], { validMessage: "", onlyOnBlur: false, wait: 300, isPhoneField: false });
const areaofInterestFieldValidationParams = { failureMessage: "This field is required" };
if (demo) {
$areaofInterest.closest(".grid-layout-col").insertAfter($productServiceInterest.closest(".grid-layout-col"));
} else {
var areaOfIntId = $areaofInterest[0].id;
if(typeof areaOfIntId != "undefined" && areaOfIntId != "") {
var label = $(`[for=${areaOfIntId}]`);
if(label.find(".elq-required").length == 0 && label.find(".required").length == 0){
label.append('<span class="elq-required">*</span>');
}
}
areaofInterestFieldValidation.add(Validate.Presence, areaofInterestFieldValidationParams);
}
var pleaseSelect = "-- Please Select --";
if (typeof areaOfInterestAutomationCustomAreaOfInterestDefault != "undefined" && areaOfInterestAutomationCustomAreaOfInterestDefault != "") {
pleaseSelect = areaOfInterestAutomationCustomAreaOfInterestDefault;
}
if (typeof labelsAsPlaceholders != "undefined" && (labelsAsPlaceholders === true || labelsAsPlaceholders === "true")) {
var label = $(`[for=${$areaofInterest.attr("id")}]`);
if (label.length > 0) {
pleaseSelect = label.text().toString().replace("*", "");
}
}
if (typeof _translateRegistrationForm != "undefined") {
pleaseSelect = _translateRegistrationForm(pleaseSelect, true);
}
$areaofInterest.html('<option value="">' + pleaseSelect + '</option>');
if (typeof amendCustomAreaOfInterestInterest == "function") {
areaOfInterestJoined = amendCustomAreaOfInterestInterest(areaOfInterestJoined);
}
for (var op in areaOfInterestJoined) {
op = areaOfInterestJoined[op];
var opLabel = op;
if (typeof _translateRegistrationForm != "undefined") {
opLabel = _translateRegistrationForm(opLabel, true);
}
var selected = [opLabel, op].includes(findGetParameter("areaofInterest1")) || [opLabel, op].includes(findGetParameter("areaofInterest")) ? " selected" : "";
$areaofInterest.append(`<option value="${op}"${selected}>${opLabel}</option>`);
}
function areaOfInterestChange() {
var demo = typeof areaOfInterestAutomationDemo !== "undefined" && areaOfInterestAutomationDemo === "true";
var val = $areaofInterest.val();
$hiddenAreaOfInterest.val(val);
if (typeof opValueMapping[val] != "undefined") {
$hiddenAreaOfInterest.val(opValueMapping[val]);
}
if (typeof defaultProductService != "undefined" && defaultProductService != "") {
if (typeof hideProductService != "undefined" && hideProductService === "true") {
return;
}
}
var text = $areaofInterest.find("option:selected").text().trim();
if (!demo) {
$productServiceInterest.closest(".grid-layout-col").show();
if (typeof val == "undefined" || val == null || val == "") {
$productServiceInterest.closest(".grid-layout-col").hide();
}
}
var selector = [];
var pleaseSelect = "-- Please Select --";
if (typeof areaOfInterestAutomationCustomProductInterestDefault != "undefined" && areaOfInterestAutomationCustomProductInterestDefault != "") {
pleaseSelect = areaOfInterestAutomationCustomProductInterestDefault;
}
if (typeof labelsAsPlaceholders != "undefined" && (labelsAsPlaceholders === true || labelsAsPlaceholders === "true")) {
var label = $(`[for=${$productServiceInterest.attr("id")}]`);
if (label.length > 0) {
pleaseSelect = label.text().toString().replace("*", "");
}
}
if (typeof _translateRegistrationForm != "undefined") {
pleaseSelect = _translateRegistrationForm(pleaseSelect, true);
}
$productServiceInterest.html('<option value="">' + pleaseSelect + '</option>');
if (typeof customSelector[val] != "undefined" || typeof customSelector[text] != "undefined") {
selector = typeof customSelector[val] != "undefined" ? customSelector[val] : customSelector[text];
} else if (bbSelector.includes(val) || bbSelector.includes(text)) {
selector = listBB;
} else if (anthologySelector.includes(val) || anthologySelector.includes(text)) {
selector = listAnthology;
}
if (demo) {
selector = [];
for (c in listAnthology) {
selector.push(listAnthology[c]);
}
for (c in listBB) {
selector.push(listBB[c]);
}
selector.sort();
}
if (typeof amendCustomAreaOfInterestProduct == "function") {
selector = amendCustomAreaOfInterestProduct(selector, val);
}
for (var op in selector) {
op = selector[op];
var opLabel = op;
if (typeof _translateRegistrationForm != "undefined") {
opLabel = _translateRegistrationForm(opLabel, true);
}
var selected = [opLabel, op].includes(findGetParameter("productServiceInterest1")) || [opLabel, op].includes(findGetParameter("productServiceInterest")) ? " selected" : "";
$productServiceInterest.append(`<option value="${op}"${selected}>${opLabel}</option>`);
}
productServiceInterestChange();
}
function productServiceInterestChange() {
var demo = typeof areaOfInterestAutomationDemo !== "undefined" && areaOfInterestAutomationDemo === "true";
var val = $productServiceInterest.val();
$hiddenProductServiceInterest.val(val);
if (typeof opValueMapping[val] != "undefined" && val != "Consulting Services") {
$hiddenProductServiceInterest.val(opValueMapping[val]);
}
if (demo) {
$areaofInterest.closest(".grid-layout-col").show();
$areaofInterest.closest(".grid-layout-col").find(".elq-required").remove();
$(".areaofInterestAutomatedFakeOption").remove();
if (typeof val != "undefined" && val != null && val != "") {
$areaofInterest.closest(".grid-layout-col").hide();
$areaofInterest.append(`<option class="areaofInterestAutomatedFakeOption" value="${val}">${val}</option>`);
$hiddenAreaOfInterest.val("");
$areaofInterest.val("");
areaofInterestFieldValidation.remove(Validate.Presence, areaofInterestFieldValidationParams);
}
else if (areaofInterestFieldValidation.validations.length == 0) {
areaofInterestFieldValidation.add(Validate.Presence, areaofInterestFieldValidationParams);
}
}
// console.log($hiddenAreaOfInterest.val());
// console.log($hiddenProductServiceInterest.val());
}
$areaofInterest.change(areaOfInterestChange);
$productServiceInterest.change(productServiceInterestChange);
if (typeof defaultWhatProblem != "undefined" && defaultWhatProblem != "") {
$("[name=whatProblem]").val(defaultWhatProblem);
$hiddenAreaOfInterest.val(defaultWhatProblem);
for (v in opValueMapping) {
if (opValueMapping[v] == defaultWhatProblem) {
defaultWhatProblem = v;
break;
}
}
if ($areaofInterest.find("[value='" + defaultWhatProblem + "']").length == 0) {
$areaofInterest.append(`<option value="${defaultWhatProblem}">${defaultWhatProblem}</option>`);
}
$areaofInterest.val(defaultWhatProblem);
}
areaOfInterestChange();
if (typeof defaultProductService != "undefined" && defaultProductService != "") {
$("[name=whatProblem]").val(defaultProductService);
$hiddenProductServiceInterest.val(defaultProductService);
if ($productServiceInterest.find("[value='" + defaultProductService + "']").length == 0) {
$productServiceInterest.append(`<option value="${defaultProductService}">${defaultProductService}</option>`);
}
$productServiceInterest.val(defaultProductService);
}
if (typeof defaultWhatProblem != "undefined" && defaultWhatProblem != "") {
if (typeof hideAreaOfInterest != "undefined" && hideAreaOfInterest === "true") {
$areaofInterest.closest(".grid-layout-col").hide();
}
}
if (typeof defaultProductService != "undefined" && defaultProductService != "") {
if (typeof hideProductService != "undefined" && hideProductService === "true") {
$productServiceInterest.closest(".grid-layout-col").hide();
}
}
}
function SetCaretAtEnd(elem) {
var elemLen = elem.value.length;
// For IE Only
if (document.selection) {
// Set focus
elem.focus();
// Use IE Ranges
var oSel = document.selection.createRange();
// Reset position to 0 & then set at end
oSel.moveStart("character", -elemLen);
oSel.moveStart("character", elemLen);
oSel.moveEnd("character", 0);
oSel.select();
} else if (elem.selectionStart || elem.selectionStart == "0") {
// Firefox/Chrome
elem.selectionStart = elemLen;
elem.selectionEnd = elemLen;
elem.focus();
}
}
var companyFiltered = $(`<div class="companyFiltered" style="display:none"></div>`);
function closestString(arr, arrSearch, str) {
str = str.toString().toLowerCase();
var orderedResult = [];
for (var i in companyList) {
var temp = sorensenDiceDistance(arrSearch[i], str);
orderedResult.push([temp * -1, arr[i]]);
}
orderedResult.sort(function (a, b) {
return a[0] - b[0];
});
return orderedResult;
}
function levenshteinDistance(str1, str2) {
var m = str1.length;
var n = str2.length;
var d = [];
for (var i = 0; i <= m; i++) {
d[i] = [];
d[i][0] = i;
}
for (var j = 0; j <= n; j++) {
d[0][j] = j;
}
for (var j = 1; j <= n; j++) {
for (var i = 1; i <= m; i++) {
if (str1[i - 1] == str2[j - 1]) {
d[i][j] = d[i - 1][j - 1];
} else {
d[i][j] = Math.min(d[i - 1][j] + 1, d[i][j - 1] + 1, d[i - 1][j - 1] + 1);
}
}
}
return d[m][n];
}
function sorensenDiceDistance(fst, snd) {
var i, j, k, map, match, ref, ref1, sub;
if (fst.length < 2 || snd.length < 2) {
return 0;
}
map = new Map;
for (i = j = 0, ref = fst.length - 2; (0 <= ref ? j <= ref : j >= ref); i = 0 <= ref ? ++j : --j) {
sub = fst.substr(i, 2);
if (map.has(sub)) {
map.set(sub, map.get(sub) + 1);
} else {
map.set(sub, 1);
}
}
match = 0;
for (i = k = 0, ref1 = snd.length - 2; (0 <= ref1 ? k <= ref1 : k >= ref1); i = 0 <= ref1 ? ++k : --k) {
sub = snd.substr(i, 2);
if (map.get(sub) > 0) {
match++;
map.set(sub, map.get(sub) - 1);
}
}
return 2.0 * match / (fst.length + snd.length - 2);
};
function filterCompany() {
companyFiltered.html("");
companyFiltered.css("display", "none");
var companyInput = $("[name=company]");
var padding = companyInput.css("padding");
var val = companyInput.val();
if (val.length > 0) {
var orderedResult = closestString(companyList, companyListLower, val);
companyFiltered.css("display", "");
if (orderedResult[0][1] != val) {
companyFiltered.append(`<div class="companyFilteredItem" onclick="selectCompany(this)">${val}</div>`);
}
for (var i = 0; i < 5; i++) {
companyFiltered.append(`<div class="companyFilteredItem" onclick="selectCompany(this)">${orderedResult[i][1]}</div>`);
}
companyFiltered.find(".companyFilteredItem").css("padding", padding);
}
}
function removeCompany() {
setTimeout(function () {
companyFiltered.css("display", "none");
}, 200);
}
function selectCompany(e) {
$("[name=company]").val($(e).text().trim());
$(".companyFiltered").css("display", "none");
}
var companyStyling = `.companyList {
position: relative;
}
.companyList .companyFiltered {
position: absolute;
width: 100%;
background-color: white;
border: 1px solid #ccc;
border-top: none;
z-index: 100;
top: 100%;
}
.companyList .companyFiltered .companyFilteredItem {
padding: 8px 20px 8px 20px;
}
.companyList .companyFiltered .companyFilteredItem:hover {
cursor: pointer;
background-color: #0090a1;
color: #fff;
}`;
$(document).ready(function () {
setTimeout(function () {
if (typeof hideFurtherEducationBasedOnCountry !== "undefined" && hideFurtherEducationBasedOnCountry === "true") {
$(document).on("change", "[name='country']", checkFurtherEducationBasedOnCountry);
checkFurtherEducationBasedOnCountry();
}
if (typeof preselectOptInIfUSAorLAC !== "undefined" && preselectOptInIfUSAorLAC === "true") {
$(document).on("change", "[name='country']", checkOptInBasedOnCountry);
checkOptInBasedOnCountry();
}
if (typeof areaOfInterestAutomation !== "undefined" && ["global", "international"].includes(areaOfInterestAutomation)) {
initAreaOfInterestAutomation(areaOfInterestAutomation);
}
if (typeof hideWhatIsYourRoleInTheDecisionMakingProcess != "undefined" && hideWhatIsYourRoleInTheDecisionMakingProcess === "true") {
$decisionMakingRole = $("[name='decisionMakingRole']");
$decisionMakingRole.closest(".grid-layout-col").hide();
}
if (typeof hideWhenDoYouPlanOnMakingaPurchase != "undefined" && hideWhenDoYouPlanOnMakingaPurchase === "true") {
$whendoyouplanonmakingapurchase1 = $("[name='whendoyouplanonmakingapurchase1']");
$whendoyouplanonmakingapurchase1.closest(".grid-layout-col").hide();
}
if (typeof enableCompanyFiltering != "undefined" && enableCompanyFiltering === "true") {
function companySearchLoad() {
// remote script has loaded
var companyInput = $("[name=company]");
// remove dublicates
companyList = companyList.filter(function (item, pos) {
return companyList.indexOf(item) == pos;
});
companyListLower = companyList.map(function (item) {
return item.toString().toLowerCase().trim();
});
companyInput.closest(".field-control-wrapper").addClass("companyList");
companyFiltered.insertAfter(companyInput);
companyInput.on('input', function () {
filterCompany();
});
companyInput.on("blur", function () {
removeCompany();
});
const injectCSS = css => {
let el = document.createElement('style');
el.type = 'text/css';
el.innerText = css;
document.head.appendChild(el);
return el;
};
injectCSS(companyStyling);
};
(function (d, script) {
script = d.createElement('script');
script.type = 'text/javascript';
script.async = true;
script.onload = companySearchLoad;
script.src = 'https://images.learn.anthology.com/Web/BlackboardInc/{4c157757-63d1-4a11-aa96-be74c43fae1a}_companyList.js';
d.getElementsByTagName('head')[0].appendChild(script);
}(document));
}
if ($("[name=jobTitleCategory1]:visible").length == 1 && $("[name=title]:visible").length == 1) {
function checkJobTitleCategory1() {
var form = $("form:not([name=translationPicklistForm])");
if (form.length == 0) {
return;
}
form = form[0];
if (typeof LiveValidationForm == "undefined" || typeof LiveValidationForm.getInstance != "function") {
return;
}
var formInstance = LiveValidationForm.getInstance(form);
var disable = false;
formInstance.fields.forEach(function name(e) {
if (typeof e.element == "undefined" || typeof e.element.name == "undefined" || typeof e.validations == "undefined") {
return;
}
if (e.element.name == "title" && e.validations.length != 0) {
disable = true;
}
})
if (disable) {
return;
}
var jobTitleCategory1 = $("[name=jobTitleCategory1]").val();
if (jobTitleCategory1.toString().toLowerCase() == "other") {
$("[name='title']").closest(".grid-layout-col").insertAfter($("[name=jobTitleCategory1]").closest(".grid-layout-col"));
$("[name='title']").closest(".grid-layout-col").show();
var titleField = window[$("[name=title]").prop('id')];
if (typeof titleField != "undefined" && typeof titleField.enable == "function") {
titleField.enable();
}
} else {
var titleField = window[$("[name=title]").prop('id')];
if (typeof titleField != "undefined" && typeof titleField.disable == "function") {
titleField.disable();
}
$("[name='title']").closest(".grid-layout-col").hide();
}
}
checkJobTitleCategory1();
$("[name=jobTitleCategory1]").change(checkJobTitleCategory1);
}
var newSrcAndContactSubscribtionFlag = $("[name=contactName]").length != 0 && $("[name=contactSrc]").length != 0 && $("[name=subscriptionSrc]").length != 0 && $("[name=subscriptionName]").length != 0 && $("[name=subscriptionDate]").length != 0;
if ($("#eloquaOptInStatus").text().toString().toLowerCase().trim() == "1") {
if (typeof disableOptInAutoHide !== 'undefined' && (disableOptInAutoHide === true || disableOptInAutoHide === 'true')) {
return;
}
if (typeof disableProgressiveProfiling === 'undefined') {
disableProgressiveProfiling = '';
}
if (disableProgressiveProfiling === 'true' || disableProgressiveProfiling === true) {
disableProgressiveProfiling = true;
} else {
disableProgressiveProfiling = false;
}
if (typeof disableProgressiveProfilingClearFields === 'undefined' || disableProgressiveProfilingClearFields === 'true' || disableProgressiveProfilingClearFields === true) {
disableProgressiveProfilingClearFields = true;
} else {
disableProgressiveProfilingClearFields = false;
}
if (typeof showAllProgressiveProfilingFields !== 'undefined' && (showAllProgressiveProfilingFields == 'true' || showAllProgressiveProfilingFields === true)) {
showAllProgressiveProfilingFields = true;
disableProgressiveProfiling = true;
} else {
showAllProgressiveProfilingFields = false;
}
if (!disableProgressiveProfiling || (disableProgressiveProfiling && !disableProgressiveProfilingClearFields)) {
$("[name=OptIn]").closest(".layout-col").addClass("d-none");
$("[name=OptIn]").closest(".layout-col").parent().css("margin-top", "20px");
$("[name=OptIn]").prop("checked", true);
window.optInInitialChecked = true;
if(newSrcAndContactSubscribtionFlag){
var formId = $("[name=contactName]").closest("form").attr("id").toString().replace("form", "");
$("[name=subscriptionSrc]").val("Form");
$("[name=subscriptionName]").val(formId);
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1;
var yyyy = today.getFullYear();
today = dd + '/' + mm + '/' + yyyy;
if(typeof moment == "function"){
today = moment().tz("America/New_York").format('DD/MM/YYYY HH:mm:ss A');
}
$("[name=subscriptionDate]").val(today);
}
}
}
if (newSrcAndContactSubscribtionFlag) {
var formId = $("[name=contactName]").closest("form").attr("id").toString().replace("form", "");
$("[name=contactName]").val(formId);
$("[name=contactSrc]").val("Form");
$("[name=OptIn]").val("1");
$("[name=OptIn]").change(function () {
if ($(this).prop("checked")) {
$("[name=subscriptionSrc]").val("Form");
$("[name=subscriptionName]").val(formId);
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth() + 1;
var yyyy = today.getFullYear();
today = dd + '/' + mm + '/' + yyyy;
if(typeof moment == "function"){
today = moment().tz("America/New_York").format('DD/MM/YYYY HH:mm:ss A');
}
$("[name=subscriptionDate]").val(today);
} else {
$("[name=subscriptionSrc]").val("");
$("[name=subscriptionName]").val("");
$("[name=subscriptionDate]").val("");
}
});
return;
}
}, 100);
});
Resources
Contact Us
Let’s discuss how we can help you build your Disability Action Plan
About Blackboard Ally
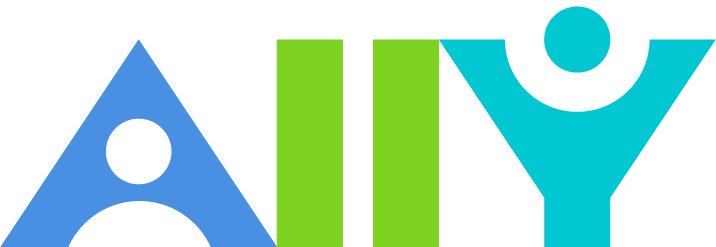
Blackboard Ally is a revolutionary, tool that integrates seamlessly into the learning management system and focuses on making digital course content more accessible. It is platform-agnostic and integrates with Moodle, Brightspace by D2L, and Canvas.
Accessible content is better content
Blackboard Ally helps institutions build a more inclusive learning environment and improve the student experience by helping them take clear control of course content with usability, accessibility and quality in mind.
Alternative formats for all learners
Improve the overall student experience with alternative formats that provide students with the choice and added flexibility that comes with a more personalised approach.
Institutional Reporting for the bigger picture
Automatically check for common accessibility issues and provide institution-wide reporting on the accessibility of your course content to efficiently prioritise your resources and tackle problems.
Instructor-Specific Feedback to Save Time & Resources
Automatically increase awareness and provide detailed insight and guidance to instructors on how to improve the accessibility of their content.
About The Author
John Doe 1
Sr. Product Manager
Company name
In arcu sapien, sagittis nec iaculis vel, ultrices vel sapien. Nullam condimentum dignissim enim. Aliquam porttitor vulputate sapien ac posuere. Sed tempor velit quam, sit amet sollicitudin lectus ultrices quis.
In arcu sapien, sagittis nec iaculis vel, ultrices vel sapien. Nullam condimentum dignissim enim. Aliquam porttitor vulputate sapien ac posuere. Sed tempor velit quam, sit amet sollicitudin lectus ultrices quis.
In arcu sapien, sagittis nec iaculis vel, ultrices vel sapien. Nullam condimentum dignissim enim. Aliquam porttitor vulputate sapien ac posuere. Sed tempor velit quam, sit amet sollicitudin lectus ultrices quis.
John Doe 2
Sr. Product Manager
Company name
In arcu sapien, sagittis nec iaculis vel, ultrices vel sapien. Nullam condimentum dignissim enim. Aliquam porttitor vulputate sapien ac posuere. Sed tempor velit quam, sit amet sollicitudin lectus ultrices quis.
In arcu sapien, sagittis nec iaculis vel, ultrices vel sapien. Nullam condimentum dignissim enim. Aliquam porttitor vulputate sapien ac posuere. Sed tempor velit quam, sit amet sollicitudin lectus ultrices quis.
In arcu sapien, sagittis nec iaculis vel, ultrices vel sapien. Nullam condimentum dignissim enim. Aliquam porttitor vulputate sapien ac posuere. Sed tempor velit quam, sit amet sollicitudin lectus ultrices quis.
“
Praesent lacus sapien, commodo id congue sed, cursus eu leo. Donec hendrerit tortor ut viverra lacinia.”
Read more
Jane Doe, M.A.
eLearning Manager
Monument University
“
Praesent lacus sapien, commodo id congue sed, cursus eu leo. Donec hendrerit tortor ut viverra lacinia.”
Read more
Jane Doe, M.A.
eLearning Manager
Monument University
Contact Us
Please fill out the following information to register for the webinar.
You have to select at least one session!
Your privacy is important to us. You can change your email preferences or unsubscribe at any time. We will only use the information you have provided to send you Anthology and Blackboard communications according to your preferences. We may share your information within the Anthology group of companies (including Blackboard Inc, and their respective subsidiaries worldwide) and with our relevant channel partners (resellers) if your organization is located in an area managed by such partners (see list here). View the Anthology Privacy Statement and Blackboard Privacy Statement for more details. For more information about the merger between Anthology and Blackboard, please view our press release.
var fixInputsFlag = false;
hideArray = ["emailAddress", "OptIn", "currentLMS1",
"onlineProgramInPlace1", "dietaryRequirements",
"firstName", "lastName", "company", "country",
"stateProv", "industry1", "jobTitleCategory1", "title", "primaryRole1",
"busPhone", "numberOfUsersANZ1", "city"
];
if (typeof formSingleColumn === 'undefined') {
formSingleColumn = '';
}
if (formSingleColumn === 'true' || formSingleColumn === true) {
formSingleColumn = true;
} else {
formSingleColumn = false;
}
function getResetNoteQuery() {
var version = $("input[name='formVersion']").val();
if (version != "2" && version != "3") {
return "#reset";
}
window.translateFormLanguage = window.translateFormLanguage ? window.translateFormLanguage : "en";
var temp = (window.translateFormLanguage == "en-uk" || window.translateFormLanguage == "en-us" || window.translateFormLanguage == "en-anz") ? "en" : window.translateFormLanguage;
return "." + temp + "-reset";
}
function getPrivacyNoteQuery() {
var version = $("input[name='formVersion']").val();
if (version != "2" && version != "3") {
return "#privacy";
}
window.translateFormLanguage = window.translateFormLanguage ? window.translateFormLanguage : "en";
var temp = (window.translateFormLanguage == "en-uk" || window.translateFormLanguage == "en-us" || window.translateFormLanguage == "en-anz") ? "en" : window.translateFormLanguage;
return "." + temp + "-privacy";
}
function numberFieldAllowdCharacters(evt) {
var allowedCharaters = ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "+", "-", "(", ")"];
if (evt.key.toString().length == 1 && (!evt.ctrlKey || evt.key != "a") && !allowedCharaters.includes(evt.key)) {
evt.preventDefault();
}
}
function fixInputs(selector) {
if (fixInputsFlag) {
return;
}
fixInputsFlag = true;
var div = document.createElement('div');
div = $(div);
div.addClass("row");
div.addClass("myContainerRow");
var target;
if (selector == "") {
selector = "form:not([name=translationPicklistForm]) input,form:not([name=translationPicklistForm]) select,form:not([name=translationPicklistForm]) textarea";
}
$(selector).each(function (index) {
$(this).closest('.layout-col').removeClass("col-sm-6");
var element = $(this).closest('.grid-layout-col');
var oldForm = false;
if (element.length == 0) {
element = $(this).closest('.individual ');
oldForm = true;
}
if ($(this).prop('type') != "hidden") {
var attrName = $(this).attr('name');
if (!formSingleColumn && attrName != 'OptIn' && $(this).prop('type') != "submit" && $(this).prop('type') != "textarea") {
if(threeColumnFormDesign){
element.addClass("col-md-custom-4");
}
else{
element.addClass("col-md-6");
}
} else {
element.addClass("col-md-12");
}
element.css('padding-left', '0');
element.css('padding-right', '0');
element.css('min-height', '0');
element.find(".layout-col").css('min-height', '0');
var toDelete = element.parent();
target = element.parent().parent();
element.appendTo(div);
toDelete.remove();
if (oldForm) {
element.addClass("form-element-layout");
if (typeof attrName === 'undefined') {
attrName = '';
}
if (hideArray.includes(attrName)) {
element.hide();
}
}
}
});
target = $("form:not([name=translationPicklistForm]) > div").filter(":not(" + getResetNoteQuery() + ")").filter(":not(" + getPrivacyNoteQuery() + ")").first();
div.appendTo(target);
if (iFrameDetection == true) {
// $("form").prop("target", "_top");
} else {
$("form.elq-form:not([name=translationPicklistForm])").append($(getPrivacyNoteQuery()).show());
$("form.elq-form:not([name=translationPicklistForm])").prepend($(getResetNoteQuery()));
if(typeof emailAddress != "undefined" && emailAddress.toString().trim() !=""){
if (!disableProgressiveProfiling || (disableProgressiveProfiling && !disableProgressiveProfilingClearFields)) {
$(getResetNoteQuery()).show();
}
}
}
}
var iFrameDetection = (window === window.parent) ? false : true;
if (!Array.prototype.find) {
Array.prototype.find = function (predicate) {
'use strict';
if (this == null) {
throw new TypeError('Array.prototype.find called on null or undefined');
}
if (typeof predicate !== 'function') {
throw new TypeError('predicate must be a function');
}
var list = Object(this);
var length = list.length >>> 0;
var thisArg = arguments[1];
var value;
for (var i = 0; i < length; i++) {
value = list[i];
if (predicate.call(thisArg, value, i, list)) {
return value;
}
}
return undefined;
};
}
if (typeof disableProgressiveProfiling === 'undefined') {
disableProgressiveProfiling = '';
}
if (disableProgressiveProfiling == 'true') {
disableProgressiveProfiling = true;
} else {
disableProgressiveProfiling = false;
}
if (typeof disableProgressiveProfilingClearFields === 'undefined') {
disableProgressiveProfilingClearFields = 'true';
}
if (disableProgressiveProfilingClearFields == 'true') {
disableProgressiveProfilingClearFields = true;
} else {
disableProgressiveProfilingClearFields = false;
}
if (typeof showAllProgressiveProfilingFields !== 'undefined' && showAllProgressiveProfilingFields == 'true') {
showAllProgressiveProfilingFields = true;
disableProgressiveProfiling = true;
}else{
showAllProgressiveProfilingFields = false;
}
var statesUsa = '<option value="" class="forceTranslate">-- Please Select --</option><option value="AA">Armed Forces Americas</option><option value="AE">Armed Forces Europe</option><option value="AK">Alaska</option><option value="AL">Alabama</option><option value="AP">Armed Forces Pacific</option><option value="AR">Arkansas</option><option value="AS">American Samoa</option><option value="AZ">Arizona</option><option value="CA">California</option><option value="CO">Colorado</option><option value="CT">Connecticut</option><option value="DC">District of Columbia</option><option value="DE">Delaware</option><option value="FL">Florida</option><option value="GA">Georgia</option><option value="GU">Guam</option><option value="HI">Hawaii</option><option value="IA">Iowa</option><option value="ID">Idaho</option><option value="IL">Illinois</option><option value="IN">Indiana</option><option value="KS">Kansas</option><option value="KY">Kentucky</option><option value="LA">Louisiana</option><option value="MA">Massachusetts</option><option value="MD">Maryland</option><option value="ME">Maine</option><option value="MI">Michigan</option><option value="MN">Minnesota</option><option value="MO">Missouri</option><option value="MS">Mississippi</option><option value="MT">Montana</option><option value="NC">North Carolina</option><option value="ND">North Dakota</option><option value="NE">Nebraska</option><option value="NH">New Hampshire</option><option value="NJ">New Jersey</option><option value="NM">New Mexico</option><option value="NV">Nevada</option><option value="NY">New York</option><option value="OH">Ohio</option><option value="OK">Oklahoma</option><option value="OR">Oregon</option><option value="PA">Pennsylvania</option><option value="PR">Puerto Rico</option><option value="RI">Rhode Island</option><option value="SC">South Carolina</option><option value="SD">South Dakota</option><option value="TN">Tennessee</option><option value="TX">Texas</option><option value="UT">Utah</option><option value="VA">Virginia</option><option value="VI">Virgin Islands</option><option value="VT">Vermont</option><option value="WA">Washington</option><option value="WI">Wisconsin</option><option value="WV">West Virginia</option><option value="WY">Wyoming</option>';
var statesAustralia = '<option value="" class="forceTranslate">-- Please Select -- </option><option value="ACT">Austl. Cap. Terr.</option><option value="NSW">New South Wales</option><option value="NT">Northern Territory</option><option value="QLD">Queensland</option><option value="SA">South Australia</option><option value="TAS">Tasmania</option><option value="VIC">Victoria</option><option value="WA">Western Australia</option>';
var statesCanada = '<option value="" class="forceTranslate">-- Please Select -- </option><option value="AB">Alberta</option><option value="BC">British Columbia</option><option value="MB">Manitoba</option><option value="NB">New Brunswick</option><option value="NF">Newfoundland</option><option value="NL">Newfoundland and Labrador</option><option value="NS">Nova Scotia</option><option value="NT">Northwest Territories</option><option value="NU">Nunavut</option><option value="ON">Ontario</option><option value="PE">Prince Edward Island</option><option value="QC">Quebec</option><option value="SK">Saskatchewan</option><option value="YT">Yukon</option><option value="ZZ">Beyond the limits of any Prov.</option>';
var statesIndia = '<option value="" class="forceTranslate">-- Please Select -- </option><option value="AP">Andhra Pradesh</option><option value="AR">Arunachal Pradesh</option><option value="AS">Assam</option><option value="BR">Bihar</option><option value="CG">Chhattisgarh</option><option value="GA">Goa</option><option value="GJ">Gujarat</option><option value="HR">Haryana</option><option value="HP">Himachal Pradesh</option><option value="JK">Jammu and Kashmir</option><option value="JH">Jharkhand</option><option value="KA">Karnataka</option><option value="KL">Kerala</option><option value="MP">Madhya Pradesh</option><option value="MH">Maharashtra</option><option value="MN">Manipur</option><option value="ML">Meghalaya</option><option value="MZ">Mizoram</option><option value="NL">Nagaland</option><option value="OD">Odisha</option><option value="PB">Punjab</option><option value="RJ">Rajasthan</option><option value="SK">Sikkim</option><option value="TN">Tamil Nadu</option><option value="TR">Tripura</option><option value="UK">Uttarakhand</option><option value="UP">Uttar Pradesh</option><option value="WB">West Bengal</option><option value="AN">Andaman and Nicobar Islands</option><option value="CH">Chandigarh</option><option value="DD">Dadra and Nagar Haveli and Daman and Diu</option><option value="DL">Delhi</option><option value="LD">Lakshadweep</option><option value="PY">Puducherry</option><option value="LA">Ladakh</option>';
var progPro = (function () {
var loopField = "";
var fieldsShown = 0;
var progShown = 0;
var country = "country";
var industry = "industry1";
var countryWithState = ["USA", "Canada", "Australia","US","CA","AU","India","IN"];
var explicitCountries = ["Canada", "Australia", "New Zealand", "Switzerland", "Austria", "Belgium", "Denmark", "Germany", "Italy", "Netherlands", "Spain"];
var optOutCountries = [
"Anguilla",
"Antigua and Barbuda",
"Argentina",
"Aruba",
"Barbados",
"Belize",
"Bermuda",
"Bolivia",
"Bonaire",
"Brazil",
"Cayman Islands",
"Chile",
"Christmas Island",
"Colombia",
"Costa Rica",
"Jamaica",
"Cuba",
"Curacao",
"Dominica",
"Dominican Republic",
"Ecuador",
"El Salvador",
"Faroe Islands",
"Falkland Islands",
"Falkland Islands (malvinas)",
"Falkland Islands (Malvinas)",
"French Guiana",
"Grenada",
"Guadeloupe",
"Guatemala",
"Guyana",
"Haiti",
"Honduras",
"Martinique",
"Mexico",
"Nicaragua",
"Panama",
"Paraguay",
"Peru",
"Saint Kitts and Nevis",
"Saint Lucia",
"Saint Martin",
"Saint Pierre and Miquelon",
"St Vincent and the Grenadines",
"The Bahamas",
"Trinidad and Tobago",
"Turks and Caicos Islands",
"Uruguay",
"Venezuela",
"Virgin Islands (British)",
"USA"
];
var whatProblem = "whatProblemAreYouTryingToSolve1";
var setRequired = function (requiredField) {
if ($("label[for=" + requiredField + "] .required").length == 0) {
var defaultValidationMessage = ($("[name=formValidMessage]").length > 0) ? $("[name=formValidMessage]").val() : "This field is required";
console.log(defaultValidationMessage + " / " + $("[name=formValidMessage]").val());
window[requiredField].add(Validate.Presence, {
failureMessage: defaultValidationMessage
});
if ($("label[for=fe128854] .elq-required").length == 0) {
$("label[for=" + requiredField + "]").append('<span class="required">*</span>');
}
console.log($(window[requiredField]).prop("name") + " is Required");
}
};
var setField = function (progField, progStage) {
try {
loopField = progField;
switch (eventType) {
case "Webinar":
var progType = progPro.contactFields.find(findProgType)[3];
break;
case "Event":
var progType = progPro.contactFields.find(findProgType)[4];
break;
case "Download":
var progType = progPro.contactFields.find(findProgType)[5];
break;
default:
var progType = progPro.contactFields.find(findProgType)[5];
break;
}
visibilityRules[progType](progField, progStage);
} catch (err) {
console.log(progStage + ": " + progField + " Not Found");
// location.reload();
}
};
var setForm = function () {
// if (progPro.formSet == true) {
// return;
// }
if (disableProgressiveProfiling) {
if (disableProgressiveProfilingClearFields) {
$(getResetNoteQuery()).hide();
$.each(progPro.contactFields, function (index, item) {
if ($("[name=" + item[0] + "]").attr("type") != "checkbox") {
$("[name=" + item[0] + "]").val("");
} else {
$("[name=" + item[0] + "]").prop("checked", false);
progPro.optInAllowed = "true";
}
});
}
}
$("[name=OptIn]").change(function () {
if ($("[name=OptIn]").prop("checked")) {
progPro.optInAllowed = "";
} else {
progPro.optInAllowed = "true";
}
});
$.each($("span.elq-required"), function (index, item) {
item = $(item);
itemParent = item.closest('.form-element-layout');
itemInput = itemParent.find("input");
if (itemInput.length == 0) {
itemInput = itemParent.find("select");
}
if (itemInput.length == 0) {
itemInput = itemParent.find("textarea");
}
if (itemInput.length == 0) {
return;
}
var settings = {
validMessage: "",
onlyOnBlur: false,
wait: 300
};
requiredField = itemInput.prop('id');
if (itemInput.length > 1) {
this.getParentElement = function (list) {
return list[list.length - 1].parentElement;
};
settings.isGroup = true;
settings.insertAfterWhatNode = this.getParentElement(itemInput);
var domVal = itemInput;
}
else{
var domVal = document.querySelector('#' + requiredField);
}
domVal = new LiveValidation(domVal, settings);
domVal.add(Validate.Presence, {
failureMessage: "This field is required"
});
console.log($(domVal).prop("name") + " is Required");
if (itemInput.prop('type') == "checkbox" && !settings.isGroup) {
itemInput.prop("required", true);
}
itemInput.addClass("elqRequiredIs");
});
fixInputs('');
progPro.formSet = true;
console.log("Set Form: " + eventType + " " + progPro.stage);
setWhatProblem();
$.each(progPro.fixedFields, function (index, item) {
progPro.setField(item, -1);
});
if (progPro.stage > 5) {
progPro.stage = 6;
}
if(showAllProgressiveProfilingFields){
$.each(progPro.contactFields, function (index, item) {
showField(item[0], -1);
});
visibilityRules.State("stateProv",-1);
}
else{
switch (progPro.stage) {
case -1:
break;
case 0:
console.log(progPro.firstVisit);
$.each(progPro.firstVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
case 1:
$.each(progPro.firstVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
case 2:
var progPrePop = 0;
$.each(progPro.secondVisit, function (index, item) {
if (($("[name=" + item + "]").val() == "") || ($("[name=" + item + "]").val() == null)) {
progPrePop++;
}
});
if (progPrePop > 0) {
$.each(progPro.secondVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
} else {
progPro.stage++;
}
case 3:
var progPrePop = 0;
$.each(progPro.thirdVisit, function (index, item) {
if (($("[name=" + item + "]").val() == "") || ($("[name=" + item + "]").val() == null)) {
progPrePop++;
}
});
if (progPrePop > 0) {
$.each(progPro.thirdVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
} else {
progPro.stage++;
}
case 4:
var progPrePop = 0;
$.each(progPro.fourthVisit, function (index, item) {
if (($("[name=" + item + "]").val() == "") || ($("[name=" + item + "]").val() == null)) {
progPrePop++;
}
});
if (progPrePop > 0) {
$.each(progPro.fourthVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
} else {
progPro.stage++;
}
case 5:
var progPrePop = 0;
$.each(progPro.fifthVisit, function (index, item) {
if (($("[name=" + item + "]").val() == "") || ($("[name=" + item + "]").val() == null)) {
progPrePop++;
}
});
if (progPrePop > 0) {
$.each(progPro.fifthVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
} else {
progPro.stage++;
}
case 6:
break;
default:
$.each(progPro.firstVisit, function (index, item) {
progPro.setField(item, progPro.stage);
});
break;
}
}
if ((eventType == "Download") && (submitButtonText == "")) {
submitButtonText = $("[name=submitButtonText]").val();
}
if (typeof _translateRegistrationForm == "function") {
submitButtonText = _translateRegistrationForm(submitButtonText);
}
if (submitButtonText != "") {
$(".submit-button").val(submitButtonText);
$("[name=submitButtonText]").val(submitButtonText);
$("[type=Submit]").val(submitButtonText);
}
$("input[type=submit]").parents('.form-element-layout').show();
$("input[type=submit]").parents('.form-element-layout').removeClass("forceHideField");
$("form:not([name=translationPicklistForm]), #wrapper").show();
if (iFrameDetection == true) {
parent.postMessage('height|' + $(document).height(), "*");
}
fixInputHeights();
if (typeof fullWebinarsUx === "function") {
try {
fullWebinarsUx();
} catch (error) {
console.error(error);
}
}
if (typeof formSetCallback === "function") {
try {
formSetCallback();
} catch (error) {
console.error(error);
}
}
$("form:not([name=translationPicklistForm]) .elq-label").each(
function (i, e) {
t = $(e).text().trim();
if (["address1","address2","address3"].includes(t)) {
$(e).closest(".grid-layout-col").hide();
}
}
)
};
var setWhatProblem = function () {
try {
if (($("[name=p]").val() != "") && ($("[name=p]").val() != null)) {
//$("[name='" + whatProblem + "']").val("");
//var myProblem = whatProblemList.find(findWhatProblem)[1];
$("[name='" + whatProblem + "']").val($("[name=p]").val());
} else {
$("[name='p']").val(defaultWhatProblem);
$("[name='" + whatProblem + "']").val(defaultWhatProblem);
}
} catch (err) {
$("[name='p']").val(defaultWhatProblem);
$("[name='" + whatProblem + "']").val(defaultWhatProblem);
}
};
var setHiddenFields = function () {
var query = window.location.search.substring(1);
var vars = query.split("&");
if (document.location.search.length) {
for (var i = 0; i < vars.length; i++) {
var pair = vars[i].split("=");
if (typeof pair[1] == "undefined") {
continue;
}
if ($("[name='" + pair[0] + "']") && (pair[1] != "")) {
var xVal = pair[1].replace(/\+/g, '%20');
xVal = xVal.replace("%A0", '');
$("[name='" + pair[0] + "']").val(decodeURIComponent(xVal));
}
}
}
if ((typeof hotLead !== 'undefined') && ((hotLead == 'true') || (hotLead == 'TRUE') || (hotLead == true))) {
$("[name='hotLead']").val("TRUE");
}
if ((typeof scoreLead !== 'undefined') && ((scoreLead == 'true') || (scoreLead == 'TRUE') || (scoreLead == true))) {
$("[name='scoreLead']").val("TRUE");
}
$("[name='eid']").val($("[name='cid']").val());
setWhatProblem();
};
var mergeHiddenFields = function (uri) {
var thisStage = parseInt($.cookie("progPro")) + 1;
if (isNaN(thisStage)) {
thisStage = 1;
}
var query = window.location.search.substring(1);
var vars = query.split("&");
for (var i = 0; i < vars.length; i++) {
var pair = vars[i].split("=");
uri = updateQueryStringParameter(uri, pair[0], pair[1]);
}
return uri + "&stage=" + thisStage;
};
var setStageCookie = function (e) {
console.log("Received: " + e.data);
if ((isNaN(e) == false) /*&& (eventType == "Download") */) {
$.cookie('progPro', e, {
expires: 180,
domain: 'blackboard.com'
});
$.cookie('progPro', e, {
expires: 180,
domain: '.anthology.com'
});
}
};
var updateQueryStringParameter = function (uri, key, value) {
var re = new RegExp("([?|&])" + key + "=.*?(&|#|$)", "i");
if (uri.match(re)) {
return uri.replace(re, '$1' + key + "=" + value + '$2');
} else {
var hash = '';
if (uri.indexOf('#') !== -1) {
hash = uri.replace(/.*#/, '#');
uri = uri.replace(/#.*/, '');
}
var separator = uri.indexOf('?') !== -1 ? "&" : "?";
return uri + separator + key + "=" + value + hash;
}
};
var setGeolookup = function () {
setField("stateProv", -1);
setField("OptIn", -1);
};
var findWhatProblem = function (problem) {
return problem[0] == $("[name='p']").val();
};
var findProgType = function (progProObj) {
return progProObj[0] == loopField;
};
var hideField = function (currentField) {
console.log("Tring to hide: " + currentField);
$("[name=" + currentField + "]").parents('.form-element-layout').hide();
$("[name=" + currentField + "]").parents('.form-element-layout').addClass("forceHideField");
if (currentField == "stateProv") {
$("[name=stateProv]").append("<option value=' '></option>");
$("[name=stateProv]").val(" ");
}
};
var showField = function (currentField, currentStage, Validation) {
console.log(currentField + " " + progShown + " " + fieldsShown + " " + currentStage);
if (currentStage == -1) {
$("[name=" + currentField + "]").parents('.form-element-layout').show();
$("[name=" + currentField + "]").parents('.form-element-layout').removeClass("forceHideField");
fieldsShown++;
if (Validation == "Required") {
setRequired($("[name=" + currentField + "]").prop('id'));
}
} else if ((fieldsShown < progPro.maxFields) && (progShown < progPro.maxProgFields)) {
$("[name=" + currentField + "]").parents('.form-element-layout').show();
$("[name=" + currentField + "]").parents('.form-element-layout').removeClass("forceHideField");
progShown++;
fieldsShown++;
if (Validation == "Required") {
setRequired($("[name=" + currentField + "]").prop('id'));
}
}
};
var resetForm = function () {
$(getResetNoteQuery()).hide();
$("span.required").remove();
// if (eventType != "Download") {
var missingFields = 0;
if (($("[name=industry1]").is(':visible') == false)) {
missingFields++;
}
if (($("[name=title]").is(':visible') == false)) {
missingFields++;
}
progShown = progShown - missingFields;
if (progShown < 0) {
progShown = 0;
}
// }
$.each(progPro.contactFields, function (index, item) {
if (($("[name=" + item[0] + "]").is(':visible') == true)) {
fieldsShown--;
}
if ($("[name=" + item[0] + "]").attr("type") != "checkbox") {
$("[name=" + item[0] + "]").val("");
} else {
$("[name=" + item[0] + "]").prop("checked", false);
progPro.optInAllowed = "true";
}
});
progPro.stage = 0;
progPro.formSet = false;
progPro.setForm();
if (iFrameDetection == true) {
parent.postMessage('height|' + $(document).height(), "*");
}
fixInputHeights();
if($("[name=OptIn]").closest(".layout-col").hasClass("d-none")){
$("[name=OptIn]").closest(".layout-col").removeClass("d-none");
$("[name=OptIn]").closest(".layout-col").parent().css("margin-top", "");
}
};
var visibilityRules = {
Required: function (currentField, currentStage) {
showField(currentField, currentStage, "Required");
},
Optional: function (currentField, currentStage) {
showField(currentField, currentStage);
},
OptionalIfBlank: function (currentField, currentStage) {
if (($("[name=" + currentField + "]").val() == "") || ($("[name=" + currentField + "]").val() == null)) {
showField(currentField, currentStage);
}
},
RequiredIfBlank: function (currentField, currentStage) {
if (($("[name=" + currentField + "]").val() == "") || ($("[name=" + currentField + "]").val() == null)) {
showField(currentField, currentStage, "Required");
}
},
Hide: function (currentField, currentStage) {
hideField(currentField, currentStage);
},
Country: function (currentField, currentStage) {
if ($("[name=country]").val() == "") {
$("[name=country]").val(countryLookup[$('[name=BbH_Country]').val()]);
}
showField(currentField, currentStage, "Required");
progPro.setField("stateProv", -1);
progPro.setField("OptIn", -1);
},
CountryIfBlank: function (currentField, currentStage) {
if (($("[name=" + currentField + "]").val() == "") || ($("[name=" + currentField + "]").val() == null)) {
if ($("[name=country]").val() == "") {
console.log($('[name=BbH_Country]').val() + " " + countryLookup[$('[name=BbH_Country]').val()]);
$("[name=country]").val(countryLookup[$('[name=BbH_Country]').val()]);
}
showField(currentField, currentStage, "Required");
progPro.setField("stateProv", -1);
progPro.setField("OptIn", -1);
}
},
OptionalIfProspect: function (currentField, currentStage) {
var useQuestion = false;
if ((currentField == "currentLMS1") && (typeof useLMSQuestion !== 'undefined') && ((useLMSQuestion == 'TRUE') || (useLMSQuestion == 'true') || (useLMSQuestion == true))) {
showField(currentField, -1);
}
if ((currentField == "onlineProgramInPlace1") && (typeof useOnlineProgramQuestion !== 'undefined') && ((useOnlineProgramQuestion == 'TRUE') || (useOnlineProgramQuestion == 'true') || (useOnlineProgramQuestion == true))) {
showField(currentField, -1);
}
if ((useQuestion == true) && (progPro.clientType == "") && (($("[name=" + currentField + "]").val() == "") || ($("[name=" + currentField + "]").val() == null))) {
showField(currentField, currentStage);
}
},
RequiredIfProspect: function (currentField, currentStage) {
var useQuestion = false;
if ((currentField == "currentLMS1") && (typeof useLMSQuestion !== 'undefined') && ((useLMSQuestion == 'TRUE') || (useLMSQuestion == 'true') || (useLMSQuestion == true))) {
showField(currentField, -1, "Required");
}
if ((currentField == "onlineProgramInPlace1") && (typeof useOnlineProgramQuestion !== 'undefined') && ((useOnlineProgramQuestion == 'TRUE') || (useOnlineProgramQuestion == 'true') || (useOnlineProgramQuestion == true))) {
showField(currentField, -1, "Required");
}
},
ReturnVisitIfBlank: function (currentField, currentStage) {
if (($("[name=" + currentField + "]").val() == "") && (progPro.stage > 1)) {
showField(currentField, currentStage);
}
},
OptIn: function (currentField, currentStage) {
var myCountry = $("[name=" + country + "]").val();
if (typeof myCountry === 'undefined') {
myCountry = "";
}
myCountry = myCountry.trim();
//$(getPrivacyNoteQuery()).hide();
if (($("[name=" + currentField + "]").not(':checked')) && (progPro.optInAllowed == "true")) {
//$(getPrivacyNoteQuery()).show();
showField(currentField, currentStage);
if ($.inArray(myCountry, optOutCountries) > -1) {
$("[name=" + currentField + "]").prop("checked", true);
} else {
$("[name=" + currentField + "]").prop("checked", false);
if(typeof optInInitialChecked != "undefined" && optInInitialChecked){
$("[name=" + currentField + "]").prop("checked", true);
}
}
$("[name=" + currentField + "]").trigger("change");
} else if (progPro.optInAllowed == "true") {
hideField(currentField, currentStage);
//$(getPrivacyNoteQuery()).hide();
}
},
State: function (currentField, currentStage) {
var myCountry = $("[name=" + country + "]").val();
myCountry = myCountry.trim();
if ($("select[name=" + currentField + "]")) {
if (statesUsa == "") {
statesUsa = $("[name=" + currentField + "]").html();
}
if (myCountry == "Australia" || myCountry == "AU") {
$("[name=" + currentField + "]").html(statesAustralia);
} else if (myCountry == "Canada" || myCountry == "CA") {
$("[name=" + currentField + "]").html(statesCanada);
} else if (myCountry == "India" || myCountry == "IN") {
$("[name=" + currentField + "]").html(statesIndia);
} else {
$("[name=" + currentField + "]").html(statesUsa);
}
if (typeof labelsAsPlaceholders != "undefined" && (labelsAsPlaceholders === true || labelsAsPlaceholders === "true")) {
if(typeof labelShrink == "function"){
labelShrink();
}
}
}
//Blank out if not Country with State
if ($.inArray(myCountry, countryWithState) == -1) {
$("[name=" + currentField + "]").val("");
hideField(currentField, currentStage);
//If Country with State, show if field is not populated
} else if (($("[name=" + currentField + "]").val() == "") || ($("[name=" + currentField + "]").val() == null)) {
showField(currentField, currentStage, "Required");
if (($("[name=" + currentField + "]").val() == "") || ($("[name=" + currentField + "]").val() == null)) {
$("[name=" + currentField + "]").val($('[name=BbH_Region]').val());
}
//Otherwise Hide
} else {
hideField(currentField, currentStage);
}
}
};
return {
setField: setField,
hideField: hideField,
showField: showField,
myList: statesUsa,
visibilityRules: visibilityRules,
setForm: setForm,
setRequired: setRequired,
setHiddenFields: setHiddenFields,
mergeHiddenFields: mergeHiddenFields,
setGeolookup: setGeolookup,
resetForm: resetForm,
setStageCookie: setStageCookie
};
})();
progPro.optInAllowed = "true";
progPro.formSet = false;
progPro.showSession = false;
progPro.stage = 0;
progPro.clientType = "";
var query = window.location.search.substring(1);
var vars = query.split("&");
for (var i = 0; i < vars.length; i++) {
var pair = vars[i].split("=");
if (pair[0] == "eventType") {
eventType = pair[1];
}
if (pair[0] == "stage") {
progPro.stage = parseInt(pair[1]);
}
if ((emailAddress == "") && (pair[0] == "em")) {
emailAddress = pair[1];
}
}
// if (eventType == "Download") {
if (progPro.stage == 0) {
progPro.stage = parseInt($.cookie("progPro")) + 1;
if (isNaN(progPro.stage) || disableProgressiveProfiling) {
progPro.stage = 1;
}
}
if(emailAddress == ""){
progPro.stage = 0;
}
console.log("Current stage: " + progPro.stage);
progPro.maxFields = 50;
progPro.maxProgFields = 50;
progPro.fixedFields = ["emailAddress", "OptIn", "currentLMS1", "onlineProgramInPlace1", "dietaryRequirements"];
progPro.firstVisit = ["firstName", "lastName", "jobTitleCategory1", "title", "company", "country", "stateProv"];
progPro.secondVisit = ["industry1", "primaryRole1", "busPhone"];
progPro.thirdVisit = ["numberOfUsersANZ1", "busPhone"];
progPro.fourthVisit = ["city", "busPhone"];
progPro.fifthVisit = ["city"];
//
// }
// if (eventType == "Event") {
//
// progPro.stage = 0;
// progPro.maxFields = 12;
// progPro.maxProgFields = 2;
// progPro.fixedFields = ["emailAddress", "firstName", "lastName", "company", "country", "stateProv", "busPhone", "OptIn", "Session", "dietaryRequirements"];
// progPro.firstVisit = ["title", "industry1", "jobTitleCategory1", "primaryRole1", "city", "numberOfUsersANZ1", "currentLMS1", "onlineProgramInPlace1"];
//
// }
// else {
//
// progPro.stage = 0;
// progPro.maxFields = 12;
// progPro.maxProgFields = 2;
// progPro.fixedFields = ["emailAddress", "firstName", "lastName", "company", "country", "stateProv", "OptIn", "Session"];
// progPro.firstVisit = ["title", "industry1", "busPhone", "jobTitleCategory1", "primaryRole1", "city", "numberOfUsersANZ1", "currentLMS1", "onlineProgramInPlace1"];
//
// }
progPro.contactFields = [
[
"emailAddress", //Form Field
'C_EmailAddress', //Eloqua Contact Field
'', //Default Value
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"firstName",
'C_FirstName',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"lastName",
'C_LastName',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"title",
'C_Title',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"company",
'C_Company',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"country",
'C_Country',
'',
'Country', //Display Rule for Webinar Form
'Country', //Display Rule for Event Form
'Country' //Display Rule for Download Form
],
[
"stateProv",
'C_State_Prov',
'',
'State', //Display Rule for Webinar Form
'State', //Display Rule for Event Form
'State' //Display Rule for Download Form
],
[
"industry1",
'C_Industry1',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"jobTitleCategory1",
'C_Job_Title_Category1',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"primaryRole1",
'C_Primary_Role1',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"busPhone",
'C_BusPhone',
'',
'Optional', //Display Rule for Webinar Form
'Optional', //Display Rule for Event Form
'Optional' //Display Rule for Download Form
],
[
"numberOfUsersANZ1",
'C_Number_of_Users__ANZ_1',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"currentLMS1",
'C_Current_LMS1',
'',
'RequiredIfProspect', //Display Rule for Webinar Form
'RequiredIfProspect', //Display Rule for Event Form
'RequiredIfProspect' //Display Rule for Download Form
],
[
"onlineProgramInPlace1",
'C_Online_Program_in_Place_1',
'',
'RequiredIfProspect', //Display Rule for Webinar Form
'RequiredIfProspect', //Display Rule for Event Form
'RequiredIfProspect' //Display Rule for Download Form
],
[
"city",
'C_City',
'',
'OptionalIfBlank', //Display Rule for Webinar Form
'OptionalIfBlank', //Display Rule for Event Form
'OptionalIfBlank' //Display Rule for Download Form
],
[
"whatProblemAreYouTryingToSolve1",
'C_What_problem_are_you_trying_to_solve_1',
'',
'Hide', //Display Rule for Webinar Form
'Hide', //Display Rule for Event Form
'Hide' //Display Rule for Download Form
],
[
"OptIn",
'C_OptIn',
'',
'OptIn', //Display Rule for Webinar Form
'OptIn', //Display Rule for Event Form
'OptIn' //Display Rule for Download Form
],
[
"Session",
'',
'',
'Required', //Display Rule for Webinar Form
'Required', //Display Rule for Event Form
'Required' //Display Rule for Download Form
],
[
"dietaryRequirements",
'',
'',
'Hide', //Display Rule for Webinar Form
'Optional', //Display Rule for Event Form
'Hide' //Display Rule for Download Form
]
];
window.onload = function () {
if (progPro.formSet == false) {
$(getResetNoteQuery()).hide();
progPro.setForm();
}
}
$(document).ready(function () {
if ($(".elq-form:not([name=translationPicklistForm])").length > 0) {
$("form:not([name=translationPicklistForm])").trigger("reset");
$("form:not([name=translationPicklistForm])").hide();
/*
$.getJSON("https://telize-v1.p.mashape.com/geoip?mashape-key=OxXupQANrAmshM2jwGNUNIx4vKeMp1iKx16jsn7daa8rE4ONZf",
function(json) {
$('[name=BbH_IP]').val(json.ip);
$('[name=BbH_Continent]').val(json.continent_code);
$('[name=BbH_Country]').val(json.country_code);
$('[name=BbH_Region]').val(json.region_code);
$('[name=BbH_PostalCode]').val(json.postal_code);
$('[name=BbH_City]').val(json.city);
if ($("[name=country]").is(":visible")) {
console.log($('[name=BbH_Country]').val() + " " + countryLookup[$('[name=BbH_Country]').val()]);
$("[name=country]").val(countryLookup[$('[name=BbH_Country]').val()]);
progPro.setGeolookup();
}
}
);
*/
$("[name='notificationEmailSubject']").val(notificationEmailSubject);
$("[name='notificationEmailRecipient']").val(notificationEmailRecipient);
$("[name='sharedListID']").val(sharedListID);
$("[name='e']").val(ConfirmationEmail);
$("[name='c']").val(ThankYouPage);
$("[name='src']").val(defaultLeadSource);
$("[name='cid']").val(sfdcCampaignId);
progPro.setHiddenFields();
setTimeout(function () {
$("[name=country]").change(function () {
progPro.setField("stateProv", -1);
progPro.setField("OptIn", -1);
fixInputHeights();
});
}, 1500);
}
if (iFrameDetection == true) {
// $("form:not([name=translationPicklistForm])").prop("target", "_top");
} else {
$("form.elq-form:not([name=translationPicklistForm])").append($(getPrivacyNoteQuery()).attr("id", "privacy"));
$("form.elq-form:not([name=translationPicklistForm])").prepend($(getResetNoteQuery()).attr("id", "reset"));
}
if ($(".elq-form:not([name=translationPicklistForm])").length == 0) {
if (emailAddress == "") {
_elqQ.push(['elqDataLookup', encodeURIComponent(VisitorLookupId), '']);
}
} else if (emailAddress == "") {
console.log('Running Unknown');
_elqQ.push(['elqDataLookup', encodeURIComponent(VisitorLookupId), '']);
} else {
console.log('Running: ' + emailAddress);
FirstLookup = false;
_elqQ.push(['elqDataLookup', encodeURIComponent(ContactLookupId), '<' + ContactUniqueField + '>' + emailAddress + '</' + ContactUniqueField + '>']);
}
$.each(progPro.contactFields, function (index, item) {
$("[name='" + this[0] + "']").parents('.form-element-layout').hide();
$("[name='" + this[0] + "']").parents('.form-element-layout').addClass("forceHideField");
});
$("#resetForm").click(function (e) {
e.preventDefault();
progPro.resetForm();
});
$("form:not([name=translationPicklistForm])").submit(function (event) {
$.each($(".elqRequiredIs"), function (index, item) {
item = $(item);
if (item.val() == "") {
item.addClass("LV_invalid_field");
} else {
item.removeClass("LV_invalid_field");
}
});
if (($('.LV_valid_field').length > 0) && ($('.LV_invalid_field:visible').length == 0) /* && (eventType == "Download") */) {
if (iFrameDetection == true) {
parent.postMessage('stage|' + progPro.stage, "*");
} else {
$.cookie('progPro', progPro.stage, {
expires: 180,
domain: 'blackboard.com'
});
$.cookie('progPro', progPro.stage, {
expires: 180,
domain: '.anthology.com'
});
}
} else if (eventType == "Download") {
return false;
}
});
progPro.setForm();
if (typeof LeadRouting === 'undefined') {
LeadRouting = "";
}
LeadRouting = LeadRouting.toLowerCase();
$('input[name="leadRouting"]').val(LeadRouting);
$("[name=busPhone]").keyup(numberFieldAllowdCharacters);
$("[name=busPhone]").keydown(numberFieldAllowdCharacters);
});
var VisitorLookupId = "753ba1c369b54b4a90656f34e15a439c"; // LOOKUP A: The ID of your Visitor Web Data Lookup
var ContactLookupId = "f52c5b076d114ec39c1dabaa33526787"; // LOOKUP B: The ID of your Contact/Datacard Web Data Lookup
var VisitorUniqueField = "V_ElqEmailAddress"; // Unique field's HTML Name from LOOKUP A (usually V_Email_Address)
var ContactUniqueField = "C_EmailAddress"; // Unique field's HTML Name from LOOKUP B (usually C_EmailAddress)
var FirstLookup = true;
// var _elqQ = _elqQ || [];
// _elqQ.push(['elqSetSiteId', '2376']);
// _elqQ.push(['elqTrackPageView']);
//
// (function() {
// function async_load() {
// var s = document.createElement('script');
// s.type = 'text/javascript';
// s.async = true;
// s.src = '//img.en25.com/i/elqCfg.min.js';
// var x = document.getElementsByTagName('script')[0];
// x.parentNode.insertBefore(s, x);
// }
// if (window.addEventListener) window.addEventListener('DOMContentLoaded', async_load, false);
// else if (window.attachEvent) window.attachEvent('onload', async_load);
// })();
function SetElqContent() {
if (typeof extraSetElqContent == 'function') {
if (extraSetElqContent()) {
return;
}
}
if (typeof webinarNameSetElqContent == 'function') {
if (webinarNameSetElqContent()) {
return;
}
}
console.log("SetElqContent");
if (this.GetElqContentPersonalizationValue) {
if (FirstLookup && this.GetElqContentPersonalizationValue.toString().includes("M_Client_Type1")) {
emailAddress = GetElqContentPersonalizationValue(VisitorUniqueField);
if (progPro.formSet == false) {
_elqQ.push(['elqDataLookup', encodeURIComponent(ContactLookupId), '<' + ContactUniqueField + '>' + emailAddress + '</' + ContactUniqueField + '>']);
FirstLookup = false;
} else {
if (typeof fullWebinarsUx === "function") {
try {
fullWebinarsUx();
} catch (error) {
console.error(error);
}
}
if (typeof formLoadedCallback === "function") {
try {
formLoadedCallback();
} catch (error) {
console.error(error);
}
}
}
} else if (this.GetElqContentPersonalizationValue.toString().includes("M_Client_Type1")) {
$.each(progPro.contactFields, function (index, item) {
item[2] = GetElqContentPersonalizationValue(item[1]);
console.log(item[2] + " " + item[1]);
if ($("[name=" + item[0] + "]").attr("type") != "checkbox") {
$("[name=" + item[0] + "]").val(item[2]);
} else if ((item[2] != "") && (item[2] != 0)) {
$("[name=" + item[0] + "]").prop("checked", true);
progPro.optInAllowed = "false";
}
});
progPro.clientType = GetElqContentPersonalizationValue('M_Client_Type1');
progPro.setForm();
if (typeof fullWebinarsUx === "function") {
try {
fullWebinarsUx();
} catch (error) {
console.error(error);
}
}
if (typeof formLoadedCallback === "function") {
try {
formLoadedCallback();
} catch (error) {
console.error(error);
}
}
}
} else {
return null;
}
}
var CollabWebinar_EventSeriesCounter = 0;
var CollabWebinar_EventSeriesCounterReceived = 0;
var CollabWebinar_EventSeriesWebinarNames = {};
var CollabWebinar_EventSeriesWebinarNames_report = false;
function webinarNameSetElqContent() {
if (this.GetElqContentPersonalizationValue) {
if (typeof GetElqContentPersonalizationValue("Event_Name1") != "undefined" && GetElqContentPersonalizationValue("Event_Name1").toString().trim() != "") {
CollabWebinar_EventSeriesCounterReceived = CollabWebinar_EventSeriesCounterReceived + 1;
var wId = GetElqContentPersonalizationValue("Event_ID1");
var temp = {
wId: wId,
eventName: GetElqContentPersonalizationValue("Event_Name1"),
maxRegistered: GetElqContentPersonalizationValue("Max_Registered1"),
registeredCounter: GetElqContentPersonalizationValue("Registered_Counter1"),
seriesName: GetElqContentPersonalizationValue("Series_Name1"),
WebinarDate: GetElqContentPersonalizationValue("Webinar_Date___Time1"),
WebinarEndDate: GetElqContentPersonalizationValue("Webinar_End_Date___Time1"),
WebinarTimezone: GetElqContentPersonalizationValue("Webinar_Timezone1"),
Recurrence: GetElqContentPersonalizationValue("Recurrence1"),
Recurring_Start_Date: GetElqContentPersonalizationValue("Recurring_Start_Date1"),
Recurring_Duration: GetElqContentPersonalizationValue("Recurring_Duration1"),
Recurring_End_Date: GetElqContentPersonalizationValue("Recurring_End_Date1")
};
if (temp.maxRegistered == "") {
temp.maxRegistered = 0;
}
if (temp.registeredCounter == "") {
temp.registeredCounter = 0;
}
temp.maxRegistered = parseInt(temp.maxRegistered);
temp.registeredCounter = parseInt(temp.registeredCounter);
if (typeof maxRegistrantsWebinarCallback == 'function') {
try {
maxRegistrantsWebinarCallback(temp);
} catch (error) {
console.error(error);
}
}
CollabWebinar_EventSeriesWebinarNames[wId] = temp;
if (CollabWebinar_EventSeriesCounterReceived == CollabWebinar_EventSeriesCounter && !CollabWebinar_EventSeriesWebinarNames_report) {
CollabWebinar_EventSeriesWebinarNames_report = true;
CollabWebinar_EventSeriesWebinarNames["endTime"] = new moment();
var secondsToFetchData = CollabWebinar_EventSeriesWebinarNames.endTime.diff(CollabWebinar_EventSeriesWebinarNames.startTime, "seconds", true);
var actionUrl = "https://content.blackboard.com/e/f2";
var data = {
url: location.href.split("?")[0],
UniqueId: new Date() / 1 + "-" + location.href.split("?")[0],
timeInSeconds: secondsToFetchData,
webinarNumber: CollabWebinar_EventSeriesCounter,
elqFormName: "Webinarinfobenchmark",
elqSiteID: "2376",
};
$.post(actionUrl, data).done(function (data) { });
}
return true;
}
}
return false;
}
var collabProgProVariablesMapping = ["CollabWebinar_EventSeries"];
for(var collabProgProVariable in collabProgProVariablesMapping){
collabProgProVariable = collabProgProVariablesMapping[collabProgProVariable];
var collabProgProVariableNoPrefix = collabProgProVariable.replace("CollabWebinar_","Webinar_");
if(typeof window[collabProgProVariableNoPrefix] !== "undefined"){
window[collabProgProVariable] = window[collabProgProVariableNoPrefix];
}
}
if (typeof EventSeries !== "undefined") {
CollabWebinar_EventSeries = EventSeries;
}
if (typeof CollabWebinar_EventSeries === "undefined") {
CollabWebinar_EventSeries = [];
}
if (typeof threeColumnFormDesign === "undefined") {
threeColumnFormDesign = false;
}
if (threeColumnFormDesign === "true") {
threeColumnFormDesign = true;
if(typeof progPro != "undefined" && typeof progPro.stage != "undefined" && progPro.stage == 3){
threeColumnFormDesign = false;
}
}
else {
threeColumnFormDesign = false;
}
if (typeof moment == "function") {
CollabWebinar_EventSeriesWebinarNames["startTime"] = new moment();
for (var i in CollabWebinar_EventSeries) {
for (var j in CollabWebinar_EventSeries[i]) {
CollabWebinar_EventSeriesCounter = CollabWebinar_EventSeriesCounter + 1;
_elqQ.push(["elqDataLookup", encodeURIComponent("ed37f4057405418bb7d4bc192b9352af"), "<Event_ID1>" + CollabWebinar_EventSeries[i][j] + "</Event_ID1>"]);
}
}
}
function webinarNameFormSuccess() {
if (typeof CollabWebinar_EventSeriesWebinarNames.endTime == "undefinde") {
return true;
}
if ($("[name='c']").val().split("?").length < 2) {
return true;
}
if ($("[name='c']").val().split("?")[1].split("se=").length < 2) {
return true;
}
if ($(".LV_invalid_field:visible").length > 0) {
return true;
}
var eventsSelected = $("[name='c']").val().split("?")[1].split("se=")[1].split("X");
var email = $("input[name=emailAddress]").val().toString().trim();
if (email == "") {
return true;
}
for (var i in eventsSelected) {
var id = eventsSelected[i].toString().trim();
var info = CollabWebinar_EventSeriesWebinarNames[id];
if (typeof info == "undefined") {
continue;
}
var actionUrl = "https://content.blackboard.com/e/f2";
if (info.Recurrence == "weekly" || info.Recurrence == "biweekly") {
var webinarTime = moment.tz(info.Recurring_End_Date, info.WebinarTimezone);
} else {
var webinarTime = moment.tz(info.WebinarEndDate, info.WebinarTimezone);
}
var pastWebinar = "yes";
if (webinarTime > moment()) {
pastWebinar = "no";
}
var data = {
eventId: id,
regId: email + "-" + id,
eventName: info.eventName,
pastWebinar: pastWebinar,
seriesName: info.seriesName,
email: email,
elqFormName: "webinarInfoBlindForm",
elqSiteID: "2376",
};
console.log(eventsSelected[i]);
console.log(CollabWebinar_EventSeriesWebinarNames[eventsSelected[i]]);
console.log(data);
$.post(actionUrl, data).done(function (data) { });
}
return true;
}
function fixInputHeights() {
if (formSingleColumn) {
return;
}
var heights = [];
var hMax = 0;
var visibleElements = [];
$("form:not([name=translationPicklistForm]) select:visible, form:not([name=translationPicklistForm]) input:visible").each(function (i, e) {
if (typeof $(e).attr("name") !== 'undefined' && $(e).attr("name") != "OptIn") {
$(e).closest(".form-element-layout").find("label").not(".elq-item-label").first().css("height", "");
}
});
if ($(window).width() < 992) {
return;
}
var visibleElements = [];
var visibleElementsLabels = [];
$("form:not([name=translationPicklistForm]) select:visible, form:not([name=translationPicklistForm]) input:visible").each(function (i, e) {
if (typeof $(e).attr("name") !== 'undefined' && $(e).attr("name") != "OptIn") {
var tempLabel = $(e).closest(".form-element-layout").find("label").not(".elq-item-label").first();
if (!visibleElementsLabels.includes(tempLabel.text())) {
var tempLabel = tempLabel;
var tH = tempLabel.outerHeight();
// var tH = tempLabel.height();
if (tH > hMax) {
hMax = tH;
}
tH = Math.ceil(tH);
tempLabel.css("height", tH);
heights.push(tH);
visibleElements.push(tempLabel);
visibleElementsLabels.push(tempLabel.text());
}
}
});
var step = 2;
if (threeColumnFormDesign) {
step = 3;
}
for (var i = 0; i + (step - 1) < visibleElements.length; i = i + step) {
var maxH = 0;
for (var j = i; j < i + step; j++) {
maxH = Math.max(maxH, heights[j]);
}
for (var j = i; j < i + step; j++) {
if (heights[j] != maxH) {
}
visibleElements[j].css("height", maxH);
}
}
}